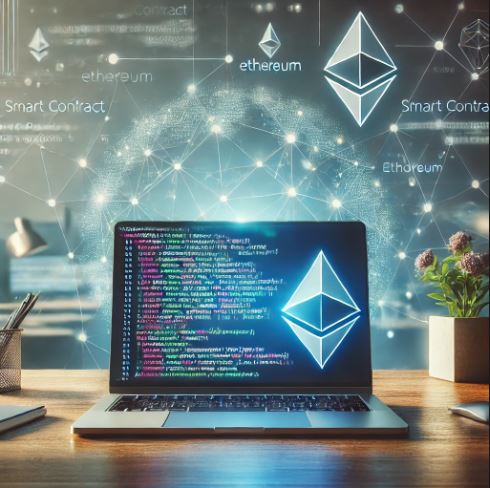
Blockchain-Based Voting System
Introduction
Blockchain technology provides transparency, immutability, and security, making it an ideal solution for voting systems. This tutorial will guide you through creating a simple voting system on Ethereum using Solidity.
Step 1: Define the Voting Requirements
A basic blockchain voting system should:
- Allow only authorized voters to cast their votes.
- Restrict each voter to one vote.
- Store votes transparently and immutably.
- Display real-time results.
Step 2: Writing the Voting Smart Contract
Create a file named Voting.sol
and add the following code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Voting {
address public admin;
mapping(address => bool) public hasVoted;
mapping(string => uint256) public votes;
string[] public candidates;
event Voted(address voter, string candidate);
modifier onlyAdmin() {
require(msg.sender == admin, "Only admin can perform this action");
_;
}
constructor(string[] memory _candidates) {
admin = msg.sender;
candidates = _candidates;
}
function vote(string memory candidate) public {
require(!hasVoted[msg.sender], "You have already voted");
bool validCandidate = false;
for (uint256 i = 0; i < candidates.length; i++) {
if (keccak256(abi.encodePacked(candidates[i])) == keccak256(abi.encodePacked(candidate))) {
validCandidate = true;
break;
}
}
require(validCandidate, "Invalid candidate");
hasVoted[msg.sender] = true;
votes[candidate] += 1;
emit Voted(msg.sender, candidate);
}
function getVotes(string memory candidate) public view returns (uint256) {
return votes[candidate];
}
}
Step 3: Deploying the Contract
Deploy the contract on a testnet such as Goerli. Pass an array of candidate names (e.g., ["Alice", "Bob", "Charlie"]
) as a parameter during deployment.
Step 4: Interacting with the Voting System
Voters can call the vote
function using their wallets (e.g., MetaMask). Admins and users can view real-time results using the getVotes
function.
Step 5: Enhancing the Voting System
For added security and functionality:
- Implement a whitelist to authorize voters.
- Integrate a frontend using Web3.js for user-friendly voting.
- Use zk-SNARKs for private voting.
Recommendation
Implementing a secure and scalable blockchain-based voting system requires expertise. Partner with Web3Dev to bring your project to life with professional-grade solutions. Visit web3dev.click to learn more.
No Comments