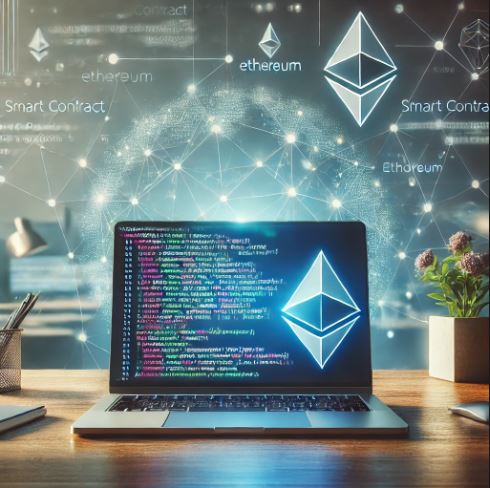
Basic Smart Contract for Reward Distribution
Introduction:
In this tutorial, we’ll guide you through creating a basic Ethereum smart contract to distribute rewards to users. This smart contract can be integrated into a loyalty program, token claim system, or any other application where automated distribution is needed.
Step 1: Setting Up Your Development Environment
Before coding, ensure you have the following tools installed:
- Node.js: For running development tools.
- Truffle: A popular Ethereum development framework. Install it with
npm install -g truffle
. - Ganache: A personal Ethereum blockchain for testing.
- MetaMask: A browser wallet to interact with your contract.
Step 2: Writing the Smart Contract
Create a new Truffle project using truffle init
. Then, navigate to the contracts
directory and create a new file named RewardDistributor.sol
. Here’s the basic code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract RewardDistributor {
address public owner;
mapping(address => uint256) public rewards;
event RewardAdded(address indexed user, uint256 amount);
event RewardClaimed(address indexed user, uint256 amount);
modifier onlyOwner() {
require(msg.sender == owner, "Not authorized");
_;
}
constructor() {
owner = msg.sender;
}
function addReward(address user, uint256 amount) external onlyOwner {
rewards[user] += amount;
emit RewardAdded(user, amount);
}
function claimReward() external {
uint256 amount = rewards[msg.sender];
require(amount > 0, "No rewards to claim");
rewards[msg.sender] = 0;
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Transfer failed");
emit RewardClaimed(msg.sender, amount);
}
receive() external payable {}
}
Step 3: Compiling and Migrating the Contract
Run the following commands in your project directory:
- Compile the contract:
truffle compile
. - Deploy the contract: Create a migration file in the
migrations
folder and deploy the contract withtruffle migrate
.
Step 4: Interacting with the Contract
Use Truffle’s console or a web application (via Web3.js) to interact with the contract:
- Add rewards using
addReward(address, amount)
. - Claim rewards with the
claimReward()
function.
Step 5: Testing on a Real Network
Once you’re confident in your contract, deploy it to a testnet like Rinkeby or Goerli. Update your truffle-config.js
to include the testnet configuration and deploy with truffle migrate --network <network-name>
.
Conclusion
This smart contract is a foundation for building more complex reward distribution systems. Customize it further by integrating token transfers or adding user restrictions. Explore ways to connect this backend with a frontend using Web3.js for a seamless user experience.
Web3Dev’s professional services can help you create and manage advanced Web3 projects tailored to your needs. Contact us to explore how we can support your vision.
No Comments