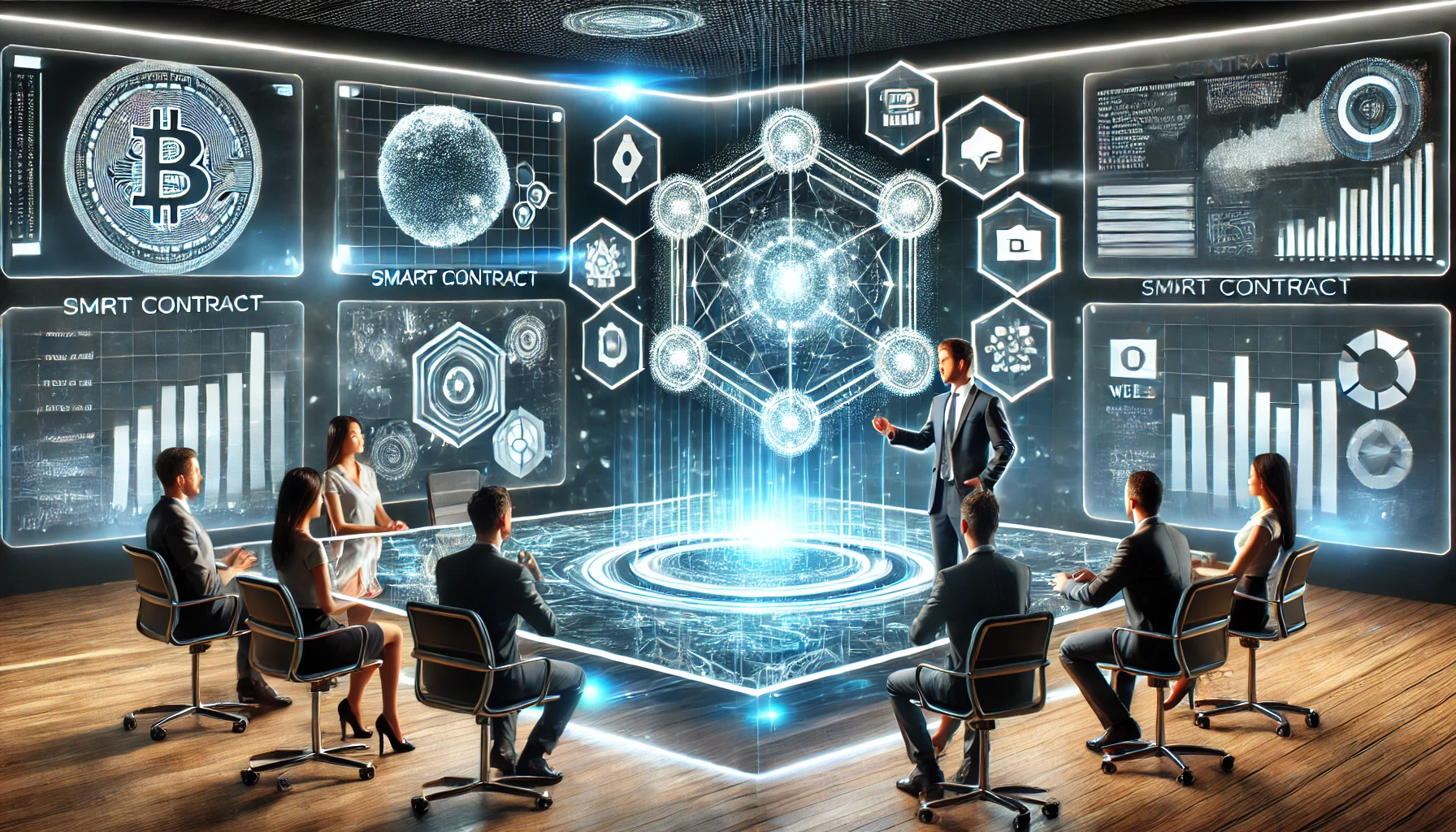
Incorrect Use of delegatecall
in Smart Contracts: A Common Vulnerability and How to Fix It
Smart contracts are the backbone of decentralized applications (dApps), but they are not immune to vulnerabilities. One of the more subtle and dangerous issues is the incorrect use of delegatecall
, which can lead to unexpected behavior, security risks, and even loss of funds. This article will explain what delegatecall
is, how it can be misused, and provide solutions to prevent vulnerabilities. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What is delegatecall
?
delegatecall
is a low-level function in Solidity that allows a contract to execute code from another contract while preserving the context (e.g., msg.sender
, msg.value
, and storage) of the calling contract. It is commonly used for proxy patterns and upgradable contracts.
However, delegatecall
is inherently risky because it allows the called contract to modify the storage of the calling contract. If not used carefully, it can lead to severe vulnerabilities.
How Does Incorrect Use of delegatecall
Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Logic {
uint256 public value;
function setValue(uint256 _value) public {
value = _value;
}
}
contract Vulnerable {
uint256 public value;
address public logicContract;
constructor(address _logicContract) {
logicContract = _logicContract;
}
function setValue(uint256 _value) public {
(bool success, ) = logicContract.delegatecall(
abi.encodeWithSignature("setValue(uint256)", _value)
);
require(success, "Delegatecall failed");
}
}
In this example, the Vulnerable
contract uses delegatecall
to execute the setValue
function from the Logic
contract. However, if the Logic
contract is malicious or contains bugs, it could modify the storage of the Vulnerable
contract in unexpected ways, leading to vulnerabilities.
The Impact of Incorrect Use of delegatecall
Incorrect use of delegatecall
can lead to:
- Storage Collisions: The called contract may overwrite critical storage variables in the calling contract.
- Unauthorized Modifications: A malicious contract could modify the state of the calling contract without permission.
- Loss of Funds: If storage variables like balances or ownership are overwritten, funds could be lost or stolen.
Solutions to Prevent Incorrect Use of delegatecall
1. Use a Structured Proxy Pattern
When using delegatecall
for upgradable contracts, follow a structured proxy pattern like the Transparent Proxy or UUPS Proxy from OpenZeppelin. These patterns ensure that storage is managed safely and upgrades are handled securely.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/proxy/TransparentUpgradeableProxy.sol";
contract MyLogic {
uint256 public value;
function setValue(uint256 _value) public {
value = _value;
}
}
contract MyProxy is TransparentUpgradeableProxy {
constructor(address _logic, address _admin, bytes memory _data)
TransparentUpgradeableProxy(_logic, _admin, _data)
{}
}
2. Isolate Storage Layout
Ensure that the storage layout of the logic contract matches the proxy contract to avoid storage collisions. Use a dedicated storage contract to define the layout.
pragma solidity ^0.8.0;
contract Storage {
uint256 public value;
}
contract Logic is Storage {
function setValue(uint256 _value) public {
value = _value;
}
}
contract Proxy is Storage {
address public logicContract;
constructor(address _logicContract) {
logicContract = _logicContract;
}
function setValue(uint256 _value) public {
(bool success, ) = logicContract.delegatecall(
abi.encodeWithSignature("setValue(uint256)", _value)
);
require(success, "Delegatecall failed");
}
}
3. Validate the Target Contract
Before using delegatecall
, validate the target contract to ensure it is trusted and secure. For example, use a whitelist of approved logic contracts.
pragma solidity ^0.8.0;
contract Secure {
uint256 public value;
address public logicContract;
mapping(address => bool) public approvedContracts;
constructor(address _logicContract) {
logicContract = _logicContract;
approvedContracts[_logicContract] = true;
}
function setValue(uint256 _value) public {
require(approvedContracts[logicContract], "Contract not approved");
(bool success, ) = logicContract.delegatecall(
abi.encodeWithSignature("setValue(uint256)", _value)
);
require(success, "Delegatecall failed");
}
}
4. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including storage collisions and unauthorized modifications. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent vulnerabilities like incorrect use of
delegatecall
. - Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments