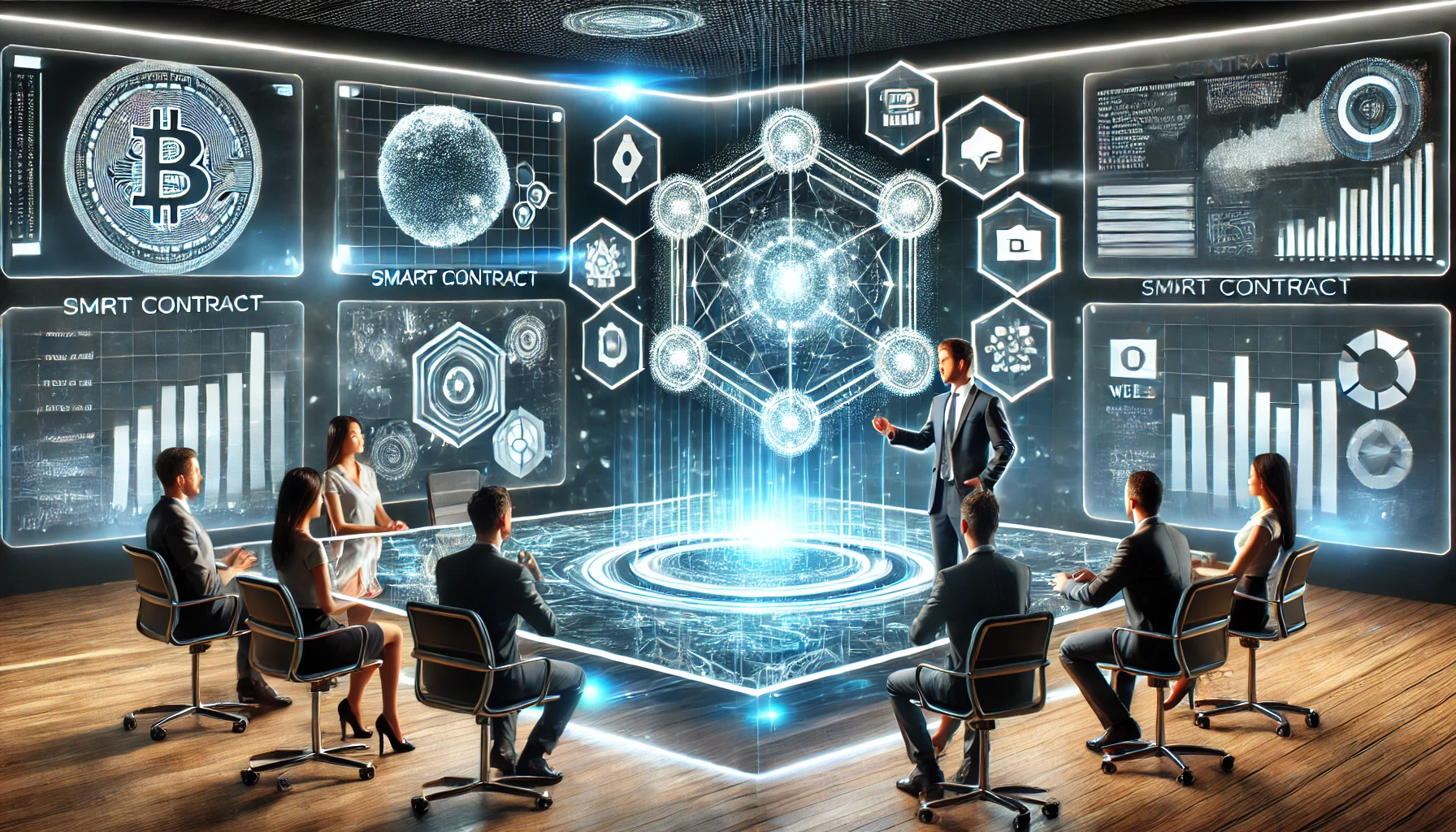
Timestamp Dependency in Smart Contracts
Smart contracts are the backbone of decentralized applications (dApps), but they are not immune to vulnerabilities. One often-overlooked issue is timestamp dependency, where a contract relies on block timestamps for critical logic. This can lead to manipulation and exploitation by miners or malicious actors. This article will explain what timestamp dependency is, how it can be exploited, and provide solutions to prevent it. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What is Timestamp Dependency?
Timestamp dependency occurs when a smart contract uses the block timestamp (block.timestamp
) for critical logic, such as determining eligibility for a function, calculating rewards, or enforcing time-based restrictions. Since miners have some control over block timestamps, they can manipulate them within a small range (typically up to 900 seconds), leading to potential vulnerabilities.
How Does Timestamp Dependency Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
uint256 public rewardEndTime;
mapping(address => uint256) public rewards;
constructor(uint256 _duration) {
rewardEndTime = block.timestamp + _duration;
}
function claimReward() public {
require(block.timestamp <= rewardEndTime, "Reward period has ended");
rewards[msg.sender] += 100; // Example reward
}
}
In this contract, the claimReward
function relies on block.timestamp
to determine if the reward period has ended. A miner could manipulate the timestamp to extend the reward period and claim additional rewards.
The Impact of Timestamp Dependency
Timestamp dependency can lead to:
- Manipulation of Contract Logic: Miners or attackers can exploit timestamp dependencies to gain unfair advantages.
- Loss of Funds: Time-based functions, such as withdrawals or rewards, can be exploited to drain funds.
- Reduced Trust: Users may lose confidence in the contract if they perceive it as unfair or exploitable.
Solutions to Prevent Timestamp Dependency
1. Use Block Numbers Instead of Timestamps
Block numbers are more reliable than timestamps because they cannot be manipulated by miners. Use block.number
for time-based logic where possible.
pragma solidity ^0.8.0;
contract Secure {
uint256 public rewardEndBlock;
mapping(address => uint256) public rewards;
constructor(uint256 _durationInBlocks) {
rewardEndBlock = block.number + _durationInBlocks;
}
function claimReward() public {
require(block.number <= rewardEndBlock, "Reward period has ended");
rewards[msg.sender] += 100; // Example reward
}
}
2. Add Buffer Periods
If you must use timestamps, add a buffer period to account for potential manipulation. For example, assume a margin of error of 900 seconds (15 minutes).
pragma solidity ^0.8.0;
contract Secure {
uint256 public rewardEndTime;
mapping(address => uint256) public rewards;
constructor(uint256 _duration) {
rewardEndTime = block.timestamp + _duration + 900; // Add buffer
}
function claimReward() public {
require(block.timestamp <= rewardEndTime, "Reward period has ended");
rewards[msg.sender] += 100; // Example reward
}
}
3. Use Oracle-Based Timestamps
For highly sensitive time-based logic, consider using a decentralized oracle like Chainlink to fetch accurate and tamper-proof timestamps.
pragma solidity ^0.8.0;
import "@chainlink/contracts/src/v0.8/interfaces/AggregatorV3Interface.sol";
contract Secure {
AggregatorV3Interface internal oracle;
uint256 public rewardEndTime;
mapping(address => uint256) public rewards;
constructor(uint256 _duration, address _oracle) {
oracle = AggregatorV3Interface(_oracle);
rewardEndTime = getCurrentTimestamp() + _duration;
}
function getCurrentTimestamp() public view returns (uint256) {
(, int256 timestamp, , , ) = oracle.latestRoundData();
return uint256(timestamp);
}
function claimReward() public {
require(getCurrentTimestamp() <= rewardEndTime, "Reward period has ended");
rewards[msg.sender] += 100; // Example reward
}
}
4. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including timestamp manipulation. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent vulnerabilities like timestamp dependency.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments