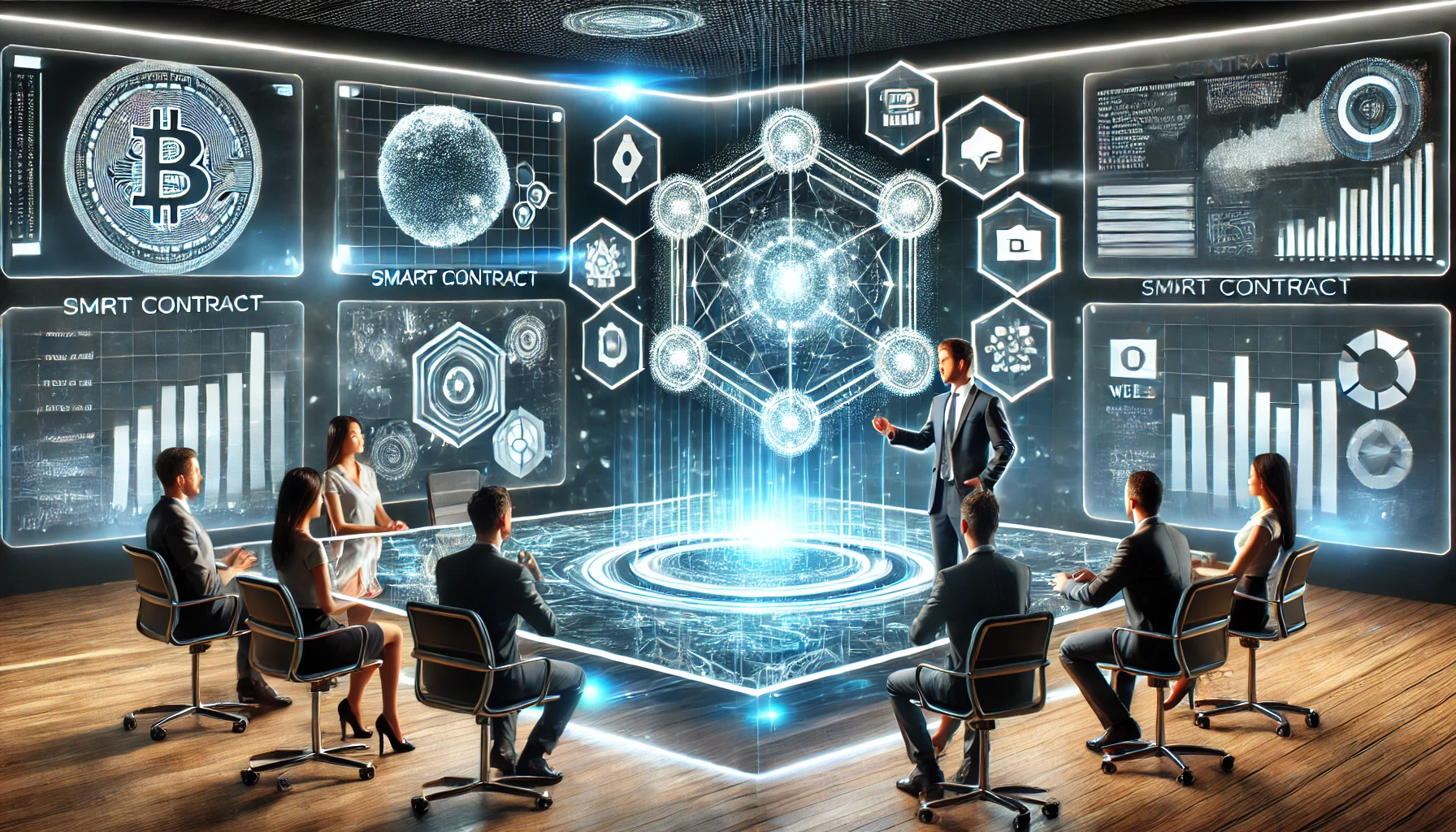
Understanding Cross-Chain Oracle Manipulation
As Web3 expands, cross-chain interoperability is becoming essential. However, cross-chain oracle manipulation is one of the most sophisticated and devastating attacks in Web3, leveraging price discrepancies between different blockchains to execute massive exploits. This type of attack targets multi-chain DeFi protocols, liquidity pools, and lending markets, often leading to losses in the hundreds of millions.
How Cross-Chain Oracle Manipulation Works
1. The Role of Oracles in Cross-Chain Finance
Oracles provide external data (such as asset prices) to smart contracts. Many DeFi platforms rely on cross-chain oracles to determine token prices for lending, borrowing, and liquidations. Attackers can manipulate these oracles to trigger unintended liquidations, execute underpriced swaps, or drain protocol reserves.
2. The Attack Process
- Find a Cross-Chain Oracle Vulnerability: The attacker identifies a price feed that is lagging or is being manipulated by a small set of nodes.
- Artificially Inflate or Deflate an Asset’s Price: By manipulating a low-liquidity market or exploiting price updates, the attacker skews the price reported by the oracle.
- Exploit DeFi Protocols on Another Chain: The manipulated price is used to liquidate massive positions, borrow overvalued assets, or execute trades at unfair prices.
- Exit Before Correction: Before arbitrage bots or governance mechanisms correct the discrepancy, the attacker cashes out.
Real-World Example: The $100M+ Attack on a Major DeFi Protocol
A real-world example occurred when an attacker exploited a price discrepancy on a low-liquidity chain and used it to take overcollateralized loans on a high-liquidity chain:
- Step 1 – Target Low-Liquidity Chain:
- The attacker found a DEX with weak price oracles on Chain A.
- They placed a large trade, pushing the price of an asset 50% higher.
- Since the oracle pulls its price from this DEX, the new price is now recorded.
- Step 2 – Trigger High-Value Arbitrage on Chain B:
- The manipulated price was used by an oracle on Chain B.
- The attacker then used the inflated price to borrow assets worth 10x their original investment.
- Step 3 – Execute and Exit:
- Before arbitrage bots could correct the price, the attacker transferred the borrowed assets to another chain and swapped them for stablecoins.
- Once liquidity providers and arbitrageurs reacted, the original price was restored—but the attacker had already withdrawn the funds.
Example of a Vulnerable Cross-Chain Oracle Implementation
pragma solidity ^0.8.0;
interface IOracle {
function getLatestPrice(address token) external view returns (uint256);
}
contract CrossChainLending {
IOracle public priceOracle;
mapping(address => uint256) public collateral;
constructor(address _oracle) {
priceOracle = IOracle(_oracle);
}
function borrow(address token, uint256 amount) external {
uint256 price = priceOracle.getLatestPrice(token);
require(collateral[msg.sender] * price / 1e18 >= amount, "Insufficient collateral");
// Issue loan
}
}
Why This is Vulnerable:
- Price Lag: If the price source is slow, attackers can manipulate it before it updates on another chain.
- Low-Liquidity Risk: The oracle pulls data from a DEX with low trading volume, making it easy to manipulate.
- Cross-Chain Delay: Any lag in data transmission between chains gives attackers time to execute their strategy.
How to Prevent Cross-Chain Oracle Exploits
1. Use Multiple Oracle Sources
Never rely on a single data source. Instead, aggregate prices from multiple sources and use a medianizer contract to prevent single-source manipulation.
contract SecureOracle {
function getMedianPrice(address token) external view returns (uint256) {
uint256 price1 = OracleA.getLatestPrice(token);
uint256 price2 = OracleB.getLatestPrice(token);
uint256 price3 = OracleC.getLatestPrice(token);
return median(price1, price2, price3);
}
}
2. Implement Time-Weighted Average Price (TWAP)
Instead of using real-time prices, use a TWAP oracle, which calculates an average price over time, making manipulation harder.
contract TWAPOracle {
uint256 public lastUpdated;
uint256 public twapPrice;
function updatePrice(uint256 newPrice) external {
require(block.timestamp > lastUpdated + 60, "Too soon to update");
twapPrice = (twapPrice + newPrice) / 2;
lastUpdated = block.timestamp;
}
}
3. Introduce Cross-Chain Security Delays
Instead of immediately acting on cross-chain price updates, introduce a security delay that allows governance mechanisms or arbitrage bots to correct manipulated prices before they are exploited.
contract DelayedOracle {
uint256 public lastPrice;
uint256 public lastUpdated;
function updatePrice(uint256 newPrice) external {
require(block.timestamp > lastUpdated + 5 minutes, "Update too soon");
lastPrice = newPrice;
lastUpdated = block.timestamp;
}
}
Conclusion: Secure Your Cross-Chain dApps
Cross-chain oracle manipulation is one of the most sophisticated and complex attack vectors in Web3. Without robust security measures, entire DeFi ecosystems can be drained in minutes.
Protect Your Project with Web3Dev
At Web3Dev, we specialize in cross-chain security, oracle integration, and DeFi protocol hardening. Our services include:
- Cross-chain security audits
- Custom oracle development
- Smart contract security hardening
- MEV and arbitrage protection
🔒 Don’t let attackers exploit your project—get in touch with Web3Dev today!
No Comments