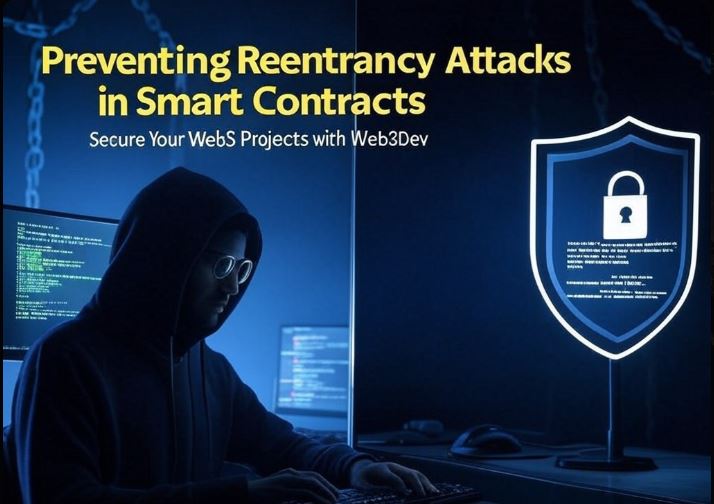
Reentrancy Attack
In the world of Web3 and decentralized applications (dApps), smart contracts are the backbone of blockchain functionality. However, they are not immune to vulnerabilities. One of the most notorious and common bugs in smart contracts is the reentrancy attack. This article will explain what a reentrancy attack is, how it can be exploited, and provide a solution to prevent it. Finally, we’ll introduce how Web3Dev, a leading Web3 development agency, can help you build secure and robust decentralized systems.
What is a Reentrancy Attack?
A reentrancy attack occurs when a malicious contract exploits a vulnerability in a target contract to repeatedly call a function before the initial function execution is complete. This is often done during a withdrawal or transfer of funds, allowing the attacker to drain the contract’s balance.
The most famous example of this vulnerability was the DAO hack in 2016, where an attacker drained over $50 million worth of Ether by exploiting a reentrancy bug.
How Does a Reentrancy Attack Work?
Here’s a simplified example of a vulnerable smart contract:
pragma solidity ^0.8.0;
contract Vulnerable {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
balances[msg.sender] -= _amount;
}
}
In this contract, the withdraw
function sends Ether to the caller before updating their balance. An attacker can exploit this by deploying a malicious contract that recursively calls the withdraw
function before the balance is updated. Here’s how:
contract Attacker {
Vulnerable public vulnerable;
constructor(address _vulnerable) {
vulnerable = Vulnerable(_vulnerable);
}
function attack() public payable {
vulnerable.deposit{value: msg.value}();
vulnerable.withdraw(msg.value);
}
receive() external payable {
if (address(vulnerable).balance >= msg.value) {
vulnerable.withdraw(msg.value);
}
}
}
The receive
function in the attacker’s contract allows it to repeatedly call the withdraw
function, draining the vulnerable contract’s funds.
The Fix: Use Checks-Effects-Interactions Pattern
To prevent reentrancy attacks, developers should follow the Checks-Effects-Interactions (CEI) pattern. This means:
- Checks: Validate all conditions before executing logic.
- Effects: Update the contract’s state (e.g., balances).
- Interactions: Interact with external contracts or addresses.
Here’s the fixed version of the withdraw
function:
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount; // Update balance first
(bool success, ) = msg.sender.call{value: _amount}("");
require(success, "Transfer failed");
}
By updating the balance before sending Ether, the contract ensures that even if the attacker recursively calls the function, their balance will already be zero, preventing further withdrawals.
Additional Security Measures
- Use Reentrancy Guards: OpenZeppelin’s
ReentrancyGuard
is a widely-used library that provides a modifier to prevent reentrancy.import "@openzeppelin/contracts/security/ReentrancyGuard.sol"; contract Secure is ReentrancyGuard { function withdraw(uint256 _amount) public nonReentrant { // Safe withdrawal logic } }
- Limit External Calls: Minimize interactions with external contracts or addresses, especially when handling funds.
- Audit Your Code: Regularly audit your smart contracts for vulnerabilities using tools like Slither, MythX, or professional auditing services.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, scalable, and innovative Web3 solutions. Our team of experienced blockchain developers and security experts can help you:
- Develop Secure Smart Contracts: We follow best practices and implement robust security measures to protect your dApps from vulnerabilities like reentrancy attacks.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential vulnerabilities before deployment.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to ensure your dApps remain secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments