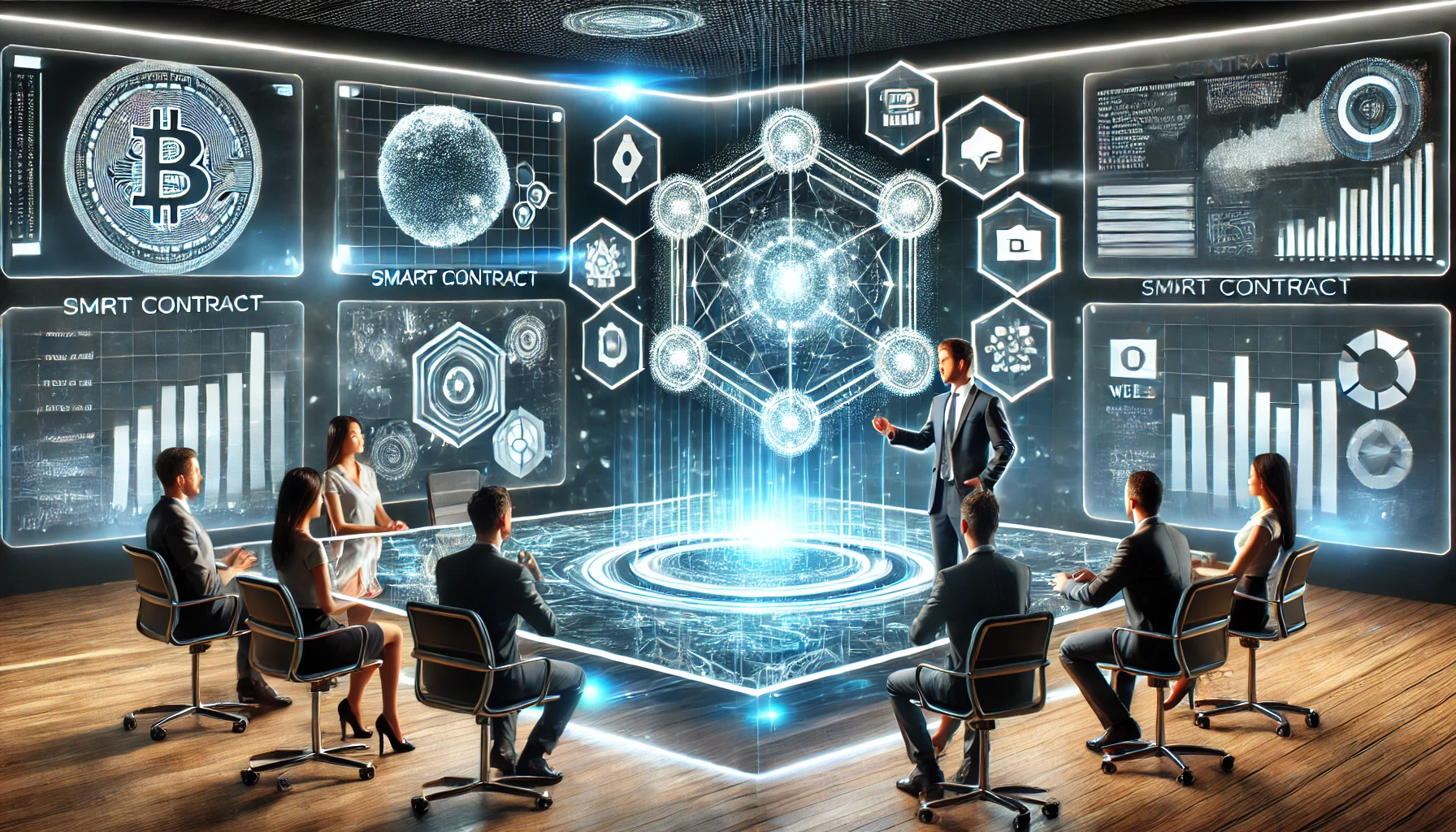
Replay Attacks in Smart Contracts: A Common Issue and How to Prevent Them
Smart contracts are the foundation of decentralized applications (dApps), but they are not immune to vulnerabilities. One often-overlooked issue is the replay attack, where an attacker reuses a valid transaction to exploit a contract multiple times. This article will explain what replay attacks are, how they work, and provide solutions to prevent them. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What is a Replay Attack?
A replay attack occurs when an attacker intercepts a valid transaction and resubmits it to the network to execute the same action multiple times. This can happen in scenarios where:
- Contracts Lack State Management: If a contract does not track whether a transaction has already been processed, an attacker can replay it.
- Cross-Chain Interactions: When transactions are valid across multiple chains (e.g., Ethereum and a fork), an attacker can replay the transaction on both chains.
Replay attacks can lead to double-spending, unauthorized actions, and loss of funds.
How Do Replay Attacks Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
payable(msg.sender).transfer(_amount);
}
}
In this contract, the withdraw
function does not track whether a withdrawal has already been processed. An attacker who intercepts a valid withdrawal transaction can replay it multiple times to drain the contract’s funds.
The Impact of Replay Attacks
Replay attacks can lead to:
- Double-Spending: Attackers can reuse transactions to withdraw funds multiple times.
- Unauthorized Actions: Replayed transactions can trigger unintended contract logic.
- Loss of Funds: Contracts may lose funds if withdrawals or transfers are replayed.
Solutions to Prevent Replay Attacks
1. Use Nonces
A nonce is a unique number used only once per transaction. By including a nonce in each transaction, you can ensure that it cannot be replayed.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
mapping(address => uint256) public nonces;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount, uint256 _nonce) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
require(nonces[msg.sender] == _nonce, "Invalid nonce");
balances[msg.sender] -= _amount;
nonces[msg.sender] += 1;
payable(msg.sender).transfer(_amount);
}
}
2. Use Chain-Specific Signatures
When dealing with cross-chain interactions, use chain-specific signatures to ensure transactions are only valid on the intended chain.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
uint256 public chainId;
constructor() {
chainId = block.chainid;
}
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount, uint256 _chainId) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
require(_chainId == chainId, "Invalid chain ID");
balances[msg.sender] -= _amount;
payable(msg.sender).transfer(_amount);
}
}
3. Implement State Tracking
Track the state of transactions to ensure they cannot be replayed. For example, use a mapping to record processed transactions.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
mapping(bytes32 => bool) public processedTransactions;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount, bytes32 _txHash) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
require(!processedTransactions[_txHash], "Transaction already processed");
balances[msg.sender] -= _amount;
processedTransactions[_txHash] = true;
payable(msg.sender).transfer(_amount);
}
}
4. Use EIP-712 for Signed Messages
EIP-712 is a standard for typed structured data hashing and signing. It helps prevent replay attacks by including domain-specific information in signed messages.
pragma solidity ^0.8.0;
contract Secure {
struct Withdraw {
address user;
uint256 amount;
uint256 nonce;
}
mapping(address => uint256) public balances;
mapping(address => uint256) public nonces;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(Withdraw calldata _withdraw, bytes calldata _signature) public {
require(balances[_withdraw.user] >= _withdraw.amount, "Insufficient balance");
require(nonces[_withdraw.user] == _withdraw.nonce, "Invalid nonce");
nonces[_withdraw.user] += 1;
balances[_withdraw.user] -= _withdraw.amount;
payable(_withdraw.user).transfer(_withdraw.amount);
}
}
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent replay attacks and other vulnerabilities.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let replay attacks or other vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments