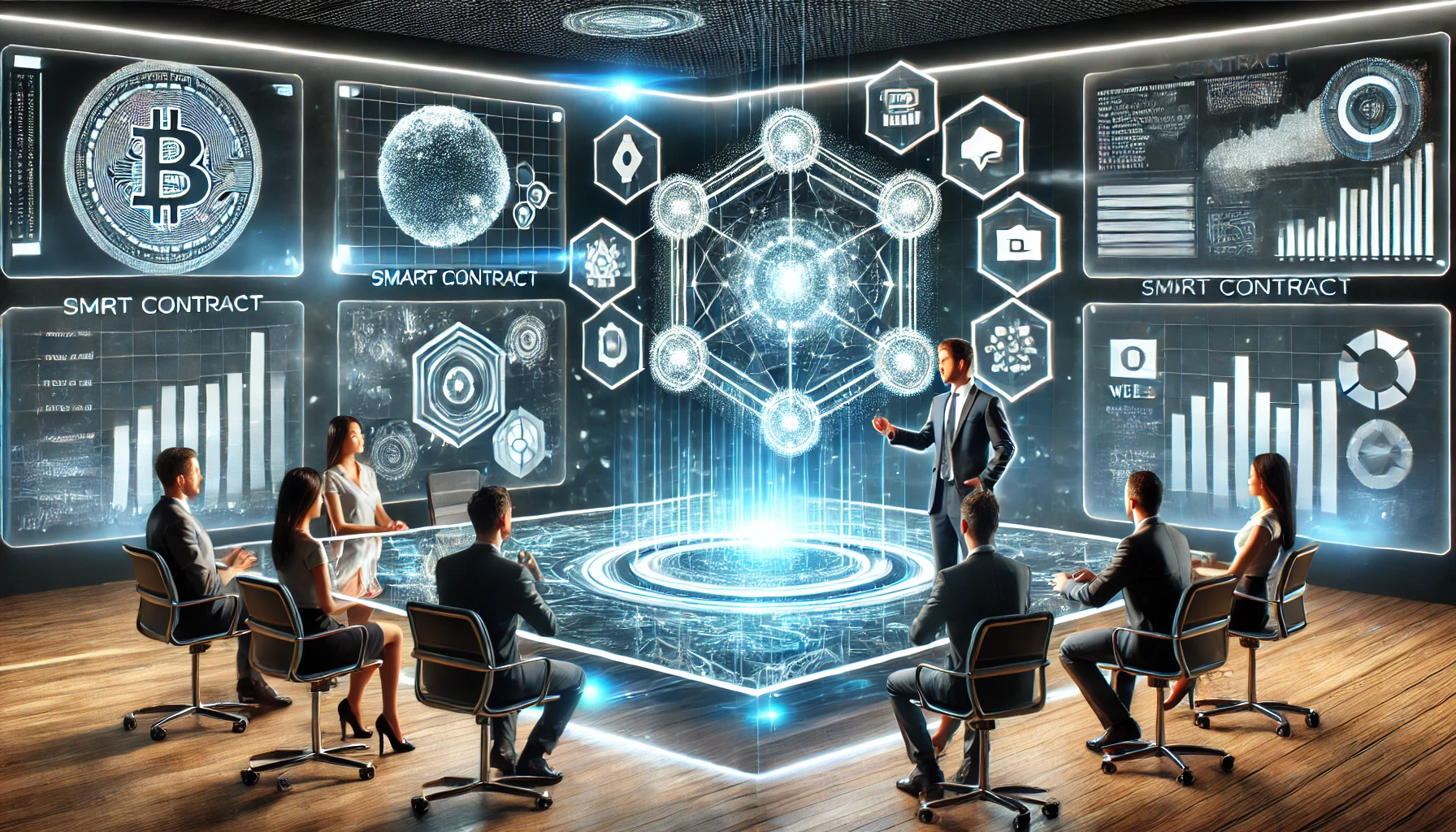
Integer Overflow and Underflow in Smart Contracts: A Common Vulnerability and How to Prevent It
Smart contracts are the foundation of decentralized applications (dApps), but they are not immune to vulnerabilities. One of the most common and potentially devastating issues is integer overflow and underflow. This article will explain what integer overflow and underflow are, how they can be exploited, and provide solutions to prevent them. Additionally, we’ll highlight how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What are Integer Overflow and Underflow?
In Solidity and many other programming languages, integers have a fixed size. For example, a uint256
can store values between 0
and 2^256 - 1
. If an operation exceeds these limits, it can result in unexpected behavior:
- Overflow: Occurs when a value exceeds the maximum limit, causing it to “wrap around” to the minimum value.
- Underflow: Occurs when a value goes below the minimum limit, causing it to “wrap around” to the maximum value.
These vulnerabilities can be exploited to manipulate contract logic, steal funds, or cause other unintended consequences.
How Do Integer Overflow and Underflow Work?
Here’s an example of a vulnerable smart contract:
pragma solidity ^0.7.0;
contract Vulnerable {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
payable(msg.sender).transfer(_amount);
}
}
In this contract, the withdraw
function is vulnerable to underflow. If a user has a balance of 5
and attempts to withdraw 10
, the subtraction balances[msg.sender] -= _amount
will result in an underflow, setting the balance to an extremely large value instead of reverting the transaction.
The Impact of Integer Overflow and Underflow
These vulnerabilities can lead to:
- Funds being stolen: Attackers can manipulate balances to withdraw more funds than they own.
- Contract logic being bypassed: Critical checks and conditions can be circumvented.
- Loss of user trust: Exploits can damage the reputation of your dApp and discourage users.
Solutions to Prevent Integer Overflow and Underflow
1. Use SafeMath Library
The SafeMath library was specifically designed to prevent integer overflow and underflow. It includes functions that automatically revert transactions if an overflow or underflow occurs.
Here’s how to use SafeMath in your contract:
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/utils/math/SafeMath.sol";
contract Secure {
using SafeMath for uint256;
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] = balances[msg.sender].add(msg.value);
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] = balances[msg.sender].sub(_amount);
payable(msg.sender).transfer(_amount);
}
}
2. Use Solidity Version 0.8.0 or Higher
Starting from Solidity version 0.8.0, the compiler automatically checks for overflow and underflow and reverts transactions if they occur. This eliminates the need for SafeMath in most cases.
Here’s an example:
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
payable(msg.sender).transfer(_amount);
}
}
3. Conduct Thorough Testing
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including potential overflow and underflow scenarios.
4. Audit Your Code
Regularly audit your smart contracts using tools like Slither, MythX, or professional auditing services to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We follow best practices and implement robust security measures to protect your dApps from vulnerabilities like integer overflow and underflow.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential vulnerabilities before deployment.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to ensure your dApps remain secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments