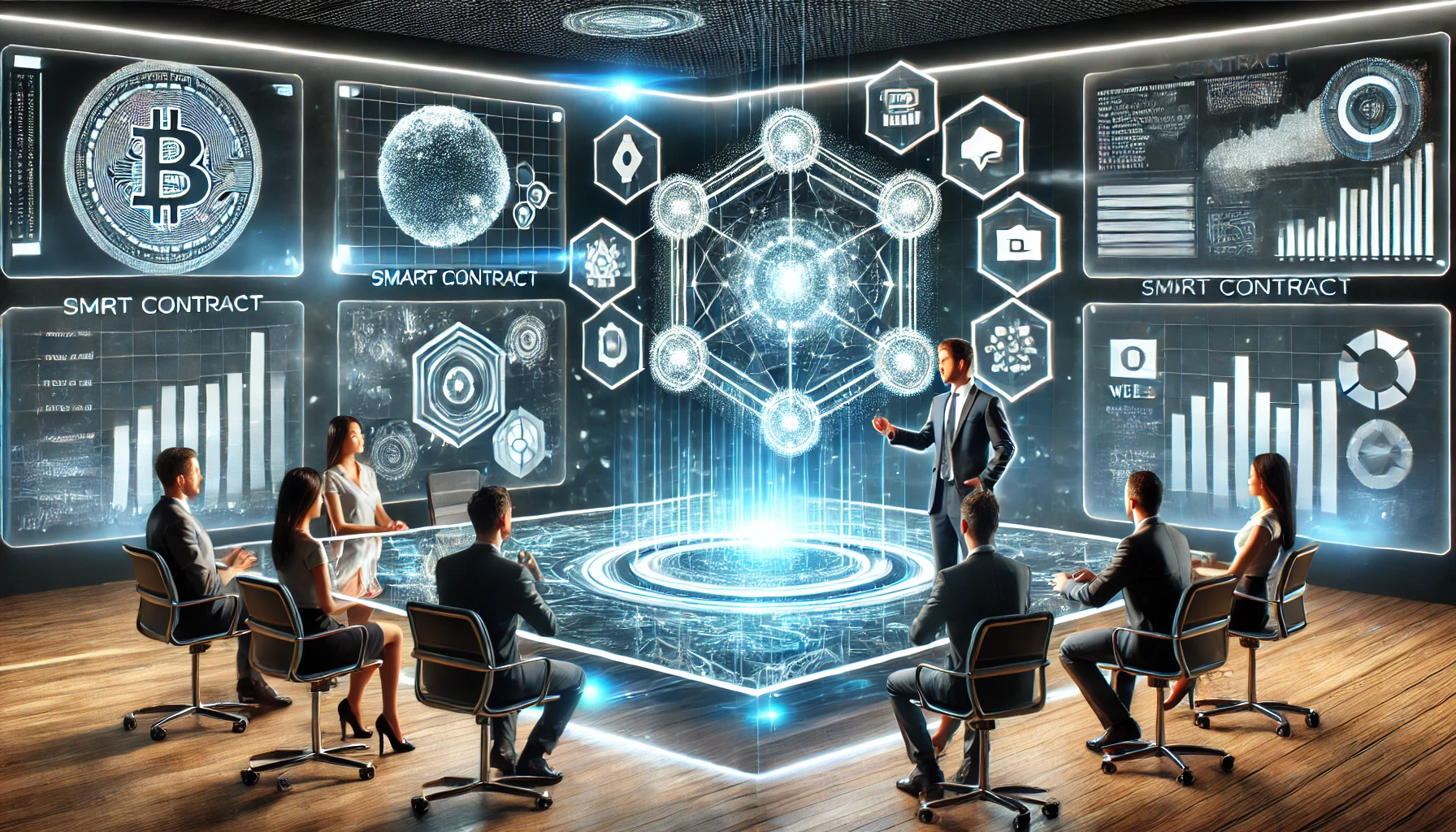
Improper Access Control in Smart Contracts
Smart contracts are the backbone of decentralized applications (dApps), but they are not immune to vulnerabilities. One of the most common and dangerous issues is improper access control, where unauthorized users can execute sensitive functions or modify critical state variables. This article will explain what improper access control is, how it can be exploited, and provide solutions to prevent it. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What is Improper Access Control?
Access control refers to the mechanisms that restrict who can execute specific functions or modify certain state variables in a smart contract. Improper access control occurs when these mechanisms are either missing or incorrectly implemented, allowing unauthorized users to perform actions they shouldn’t be able to.
Common examples include:
- Functions that lack access control modifiers (e.g.,
onlyOwner
). - Misconfigured role-based access control (RBAC) systems.
- Publicly exposed functions that should be restricted.
How Does Improper Access Control Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
address public owner;
uint256 public sensitiveValue;
constructor() {
owner = msg.sender;
}
function setSensitiveValue(uint256 _value) public {
sensitiveValue = _value; // No access control
}
}
In this contract, the setSensitiveValue
function is publicly accessible, meaning anyone can call it and modify the sensitiveValue
variable. This is a clear example of improper access control.
The Impact of Improper Access Control
Improper access control can lead to:
- Unauthorized Modifications: Attackers can change critical state variables, such as ownership or balances.
- Loss of Funds: Sensitive functions, like withdrawals or transfers, can be exploited to steal funds.
- Disruption of Services: Attackers can disrupt the functionality of the contract, rendering it unusable.
Solutions to Prevent Improper Access Control
1. Use Access Control Modifiers
Implement access control modifiers to restrict sensitive functions to authorized users. For example, use OpenZeppelin’s Ownable
or AccessControl
libraries.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/access/Ownable.sol";
contract Secure is Ownable {
uint256 public sensitiveValue;
function setSensitiveValue(uint256 _value) public onlyOwner {
sensitiveValue = _value;
}
}
2. Implement Role-Based Access Control (RBAC)
For more complex access control requirements, use OpenZeppelin’s AccessControl
library to define and manage roles.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/access/AccessControl.sol";
contract Secure is AccessControl {
bytes32 public constant ADMIN_ROLE = keccak256("ADMIN_ROLE");
uint256 public sensitiveValue;
constructor() {
_setupRole(ADMIN_ROLE, msg.sender);
}
function setSensitiveValue(uint256 _value) public onlyRole(ADMIN_ROLE) {
sensitiveValue = _value;
}
}
3. Validate Function Parameters
Ensure that function parameters are validated to prevent unauthorized actions. For example, check that the caller is the owner or has the required role.
pragma solidity ^0.8.0;
contract Secure {
address public owner;
uint256 public sensitiveValue;
constructor() {
owner = msg.sender;
}
function setSensitiveValue(uint256 _value) public {
require(msg.sender == owner, "Unauthorized");
sensitiveValue = _value;
}
}
4. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including unauthorized access attempts. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent vulnerabilities like improper access control.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments