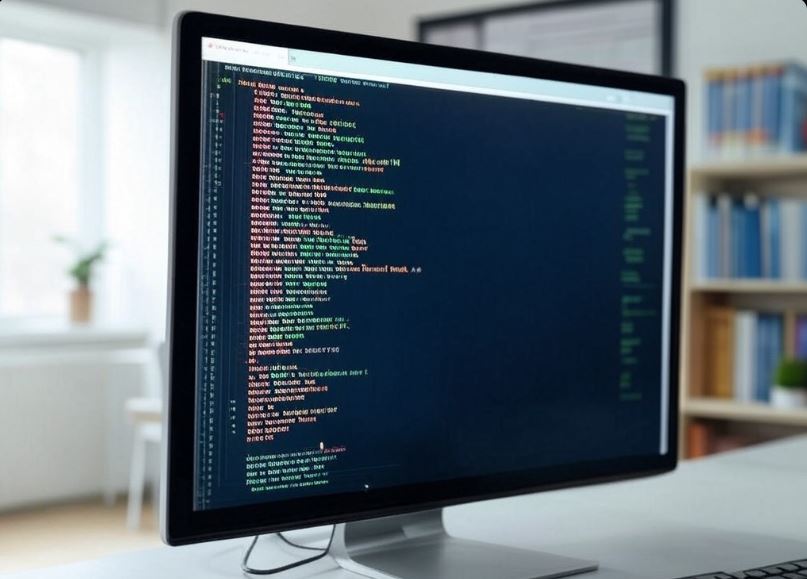
Create Your Own Token on Ethereum
Creating your own token on the Ethereum blockchain can be for various purposes, like funding a project via an Initial Coin Offering (ICO), creating a governance token for a DAO, or even for artistic expression. Here’s how you can mint your own ERC-20 token:
Step 1: Understanding Token Standards
- ERC-20: The most common standard for fungible tokens on Ethereum. It includes functions like total supply, balance, transfer, and allowance.
- Other Standards: Consider ERC-721 for non-fungible tokens or ERC-1155 for multi-token types if your use case differs.
Step 2: Set Up Your Development Environment
- Install Necessary Software:
- Node.js and npm: For running JavaScript and managing project dependencies.
- Truffle Suite: A development framework for Ethereum, including Truffle for smart contract compilation and migration.
- Solidity: The programming language for Ethereum smart contracts.
- Environment: Set up an IDE like Visual Studio Code or Remix for coding.
Step 3: Write Your Token Contract
- Basic Structure: Here’s a simple ERC-20 contract in Solidity:
solidity
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC20/ERC20.sol";
contract MyToken is ERC20 {
constructor(uint256 initialSupply) ERC20("MyToken", "MTK") {
_mint(msg.sender, initialSupply);
}
}
- Customization: Modify this contract to add features like minting control, burn functions, or custom logic.
Step 4: Deploy Your Contract
- Compile: Use Truffle or Remix to compile your contract.
- Test: Write and run tests locally to ensure your token behaves as intended.
- Deployment:
- Connect to Ethereum Network: You can deploy on testnets like Rinkeby for practice or the mainnet for real-world use.
- Use Truffle: For deployment, create a migration script:
javascript
const MyToken = artifacts.require("MyToken");
module.exports = function(deployer) {
const initialSupply = web3.utils.toWei('1000000', 'ether'); // Example supply
deployer.deploy(MyToken, initialSupply);
};
- Deploy: Run truffle migrate –network {networkName}.
Step 5: Interact with Your Token
- Wallet: Use a wallet like MetaMask to interact with your token:
- Add Custom Token: Enter your token’s contract address to view and manage it in your wallet.
- DApps: You can also create or use decentralized applications (DApps) for token management or distribution.
Step 6: Token Distribution
- Airdrop: If you’re distributing tokens, consider methods like airdrops or public sales.
- Lockup: For project funding, you might implement vesting schedules to lock up tokens.
Step 7: Maintain and Update
- Security Audits: Before public use, get your smart contract audited.
- Updates: If you need to update functionality, you might need to deploy a new version or use upgradeable contracts.
Creating your own token opens numerous possibilities in the blockchain ecosystem. However, it comes with responsibilities regarding security, compliance, and community management.
Need Professional Assistance?
If token creation feels overwhelming or if you need expert advice on smart contract development, security, or compliance, Web3Dev is here to help. They can guide you through the process, ensure your token meets industry standards, and help with ongoing maintenance. Contact us.
No Comments