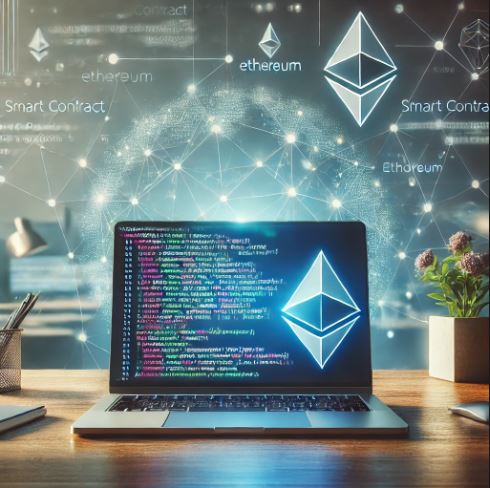
Decentralized File Storage System Using IPFS and Ethereum
Introduction
Decentralized file storage ensures security, transparency, and resistance to censorship. By combining IPFS (InterPlanetary File System) for storage and Ethereum for tracking file ownership, you can build a secure and decentralized file storage solution. This tutorial walks you through the process.
Step 1: Setting Up the Environment
Install the necessary tools:
- IPFS CLI: Install from ipfs.io.
- Node.js: For running the backend.
- Metamask: To interact with Ethereum.
Step 2: Uploading Files to IPFS
IPFS stores files in a distributed manner. To upload a file:
- Start the IPFS daemon:
ipfs daemon
- Add a file to IPFS:
ipfs add <filename>
This command returns a unique Content Identifier (CID), which you’ll use to access the file.
Step 3: Writing the Ethereum Smart Contract
The smart contract will record file ownership. Create a file named FileStorage.sol
:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract FileStorage {
struct File {
string cid;
string name;
address owner;
uint256 timestamp;
}
mapping(string => File) public files;
event FileUploaded(string cid, string name, address owner, uint256 timestamp);
function uploadFile(string memory cid, string memory name) public {
require(bytes(files[cid].cid).length == 0, "File already exists");
files[cid] = File(cid, name, msg.sender, block.timestamp);
emit FileUploaded(cid, name, msg.sender, block.timestamp);
}
function getFile(string memory cid) public view returns (File memory) {
return files[cid];
}
}
Step 4: Deploying the Contract
Deploy the contract on a testnet such as Goerli. Use tools like Remix or Hardhat for deployment.
Step 5: Integrating IPFS and Ethereum
Create a Node.js backend or frontend using libraries like Web3.js and IPFS HTTP client. The process involves:
- Uploading files to IPFS to get the CID.
- Using the CID to call the
uploadFile
function in the smart contract. - Retrieving file details using the
getFile
function.
Step 6: Enhancing the System
For a robust file storage system, you can:
- Implement access control to restrict file viewing.
- Add encryption for secure file storage.
- Enable file sharing between users.
Recommendation
Decentralized file storage can revolutionize how we manage data, but building a scalable and secure solution can be complex. Leverage Web3Dev’s expertise to create professional-grade decentralized storage systems. Visit web3dev.click for more information.
No Comments