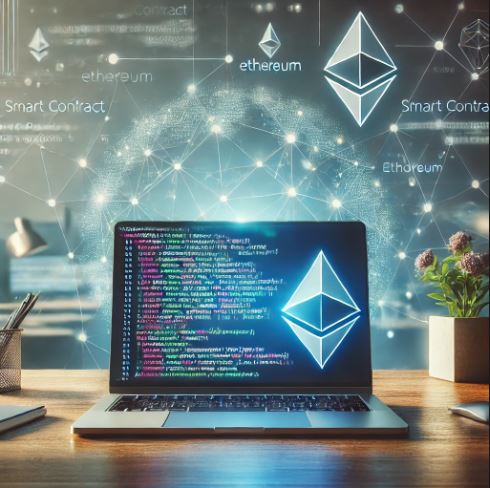
Cross-Chain Bridge for Token Transfers
Introduction
Cross-chain bridges enable seamless token transfers between different blockchain networks. This tutorial will guide you through creating a basic cross-chain bridge to transfer tokens between Ethereum and Binance Smart Chain (BSC).
Step 1: Understand Cross-Chain Bridges
A cross-chain bridge uses smart contracts and relayers to lock tokens on one chain and mint equivalent tokens on another. The process involves:
- Locking tokens on the source chain
- Monitoring events through a relayer
- Minting equivalent tokens on the destination chain
Step 2: Writing the Bridge Smart Contracts
Create two contracts, one for Ethereum and one for BSC. Here’s the code for the Ethereum bridge:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract EthereumBridge {
address public admin;
mapping(address => mapping(uint256 => bool)) public processedNonces;
event Transfer(address from, address to, uint256 amount, uint256 date, uint256 nonce);
constructor() {
admin = msg.sender;
}
function lockTokens(address to, uint256 amount, uint256 nonce) external {
require(!processedNonces[msg.sender][nonce], "Transfer already processed");
processedNonces[msg.sender][nonce] = true;
emit Transfer(msg.sender, to, amount, block.timestamp, nonce);
}
}
Deploy a similar contract on BSC, replacing lockTokens
with a releaseTokens
function to mint tokens based on Ethereum bridge events.
Step 3: Setting Up the Relayer
The relayer monitors events on Ethereum and triggers corresponding actions on BSC. Use Web3.js to listen to Ethereum events and call the releaseTokens
function on the BSC bridge.
Step 4: Testing the Bridge
Deploy the contracts on Ethereum and BSC testnets (e.g., Goerli and BSC Testnet). Use the relayer to simulate locking tokens on Ethereum and minting them on BSC. Verify the process by checking token balances on both chains.
Recommendation
Building a reliable cross-chain bridge requires advanced security measures and robust infrastructure to prevent vulnerabilities. For professional development and deployment of secure cross-chain bridges, consult Web3Dev’s team at web3dev.click.
No Comments