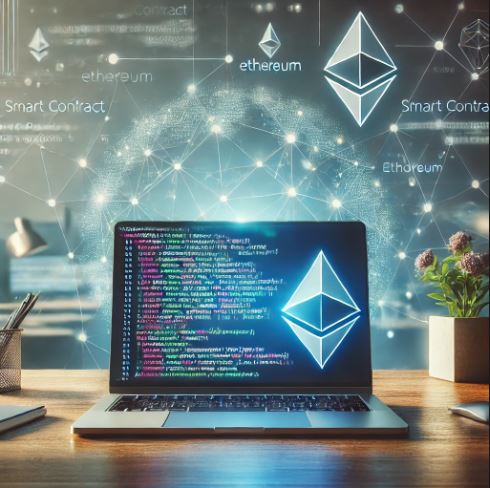
Introduction
A decentralized marketplace allows users to buy and sell goods or services without relying on a central authority. By leveraging smart contracts, you can create a trustless and transparent platform. This tutorial walks you through building a basic decentralized marketplace on Ethereum.
Step 1: Define Marketplace Features
The marketplace will have the following basic features:
- Sellers can list items for sale with a name, price, and description.
- Buyers can purchase items by sending Ether.
- Transactions are recorded on the blockchain.
Step 2: Writing the Marketplace Smart Contract
Create a file named Marketplace.sol
with the following code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract Marketplace {
struct Item {
uint256 id;
string name;
string description;
uint256 price;
address payable seller;
bool sold;
}
uint256 public itemCount;
mapping(uint256 => Item) public items;
event ItemListed(uint256 id, string name, uint256 price, address seller);
event ItemSold(uint256 id, address buyer, uint256 price);
function listItem(string memory name, string memory description, uint256 price) public {
require(price > 0, "Price must be greater than zero");
itemCount++;
items[itemCount] = Item(itemCount, name, description, price, payable(msg.sender), false);
emit ItemListed(itemCount, name, price, msg.sender);
}
function buyItem(uint256 id) public payable {
Item storage item = items[id];
require(id > 0 && id <= itemCount, "Item does not exist");
require(!item.sold, "Item already sold");
require(msg.value == item.price, "Incorrect payment amount");
item.seller.transfer(msg.value);
item.sold = true;
emit ItemSold(id, msg.sender, item.price);
}
}
Step 3: Deploying the Contract
Deploy the contract on a testnet such as Goerli. Sellers can call listItem
to add items to the marketplace, while buyers can call buyItem
to purchase items.
Step 4: Creating a User Interface
Use Web3.js or Ethers.js to connect your contract with a frontend application. Create a user-friendly interface for listing and buying items.
Step 5: Enhancing the Marketplace
Consider adding advanced features such as:
- A reputation system for buyers and sellers.
- Support for multiple payment methods, including tokens.
- Escrow functionality for added security.
Recommendation
Building a fully functional decentralized marketplace requires expertise in blockchain development and UI/UX design. For end-to-end support, consult Web3Dev’s professional services at web3dev.click. Let us help you create a secure and user-friendly platform.
No Comments