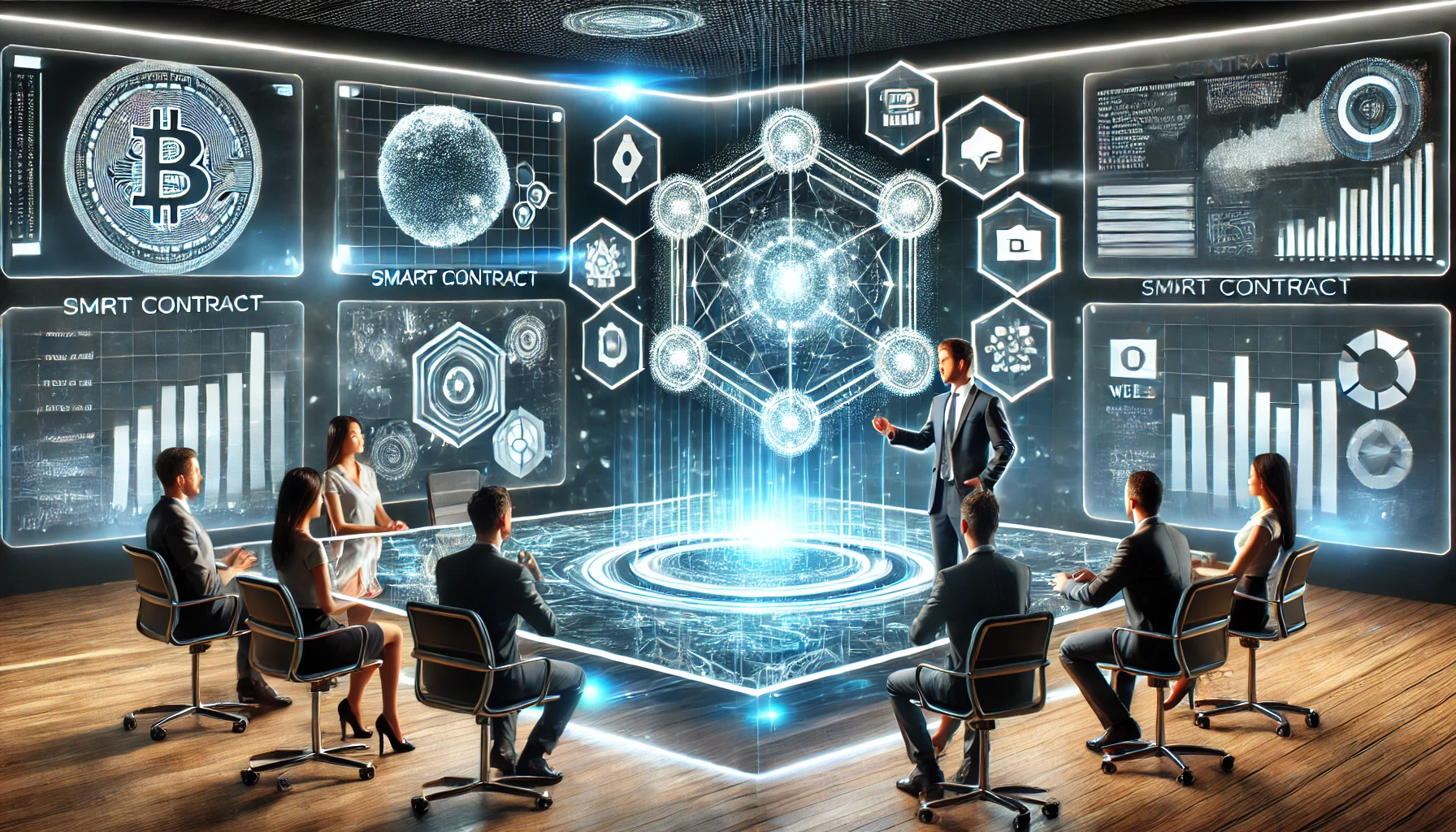
Understanding the Complexity of Maximum Extractable Value (MEV) Front-Running
One of the most intricate yet often overlooked issues in Web3 development is Maximum Extractable Value (MEV) front-running attacks. These attacks exploit the inherent transparency of blockchain transactions, allowing malicious actors to manipulate the order of transactions in a block for profit. MEV has become a growing issue, especially on Ethereum and other EVM-compatible networks, leading to significant financial losses.
How MEV Front-Running Works
MEV front-running occurs when a bot or miner/validator monitors the mempool (a pool of pending transactions) and prioritizes its own transactions over others for profit. The most common form of front-running is known as a sandwich attack, which affects decentralized exchanges (DEXs) and Automated Market Makers (AMMs).
Example of an MEV Sandwich Attack
- Victim submits a large swap on a DEX (e.g., swapping ETH for a token).
- Attacker sees the transaction in the mempool and quickly submits a buy order before the victim’s transaction is executed.
- Victim’s trade is executed at a higher price due to slippage.
- Attacker sells the token immediately after the victim’s trade, profiting from the artificial price increase.
Code Example of MEV Bot Exploiting Unprotected Transactions
// Example of a vulnerable swap function
pragma solidity ^0.8.0;
interface IUniswapV2Router {
function swapExactETHForTokens(
uint amountOutMin,
address[] calldata path,
address to,
uint deadline
) external payable;
}
contract VulnerableDEXTrade {
IUniswapV2Router public uniswapRouter;
constructor(address _router) {
uniswapRouter = IUniswapV2Router(_router);
}
function swapETHForTokens(address token, uint amountOutMin) external payable {
address[] memory path = new address[](2);
path[0] = 0xC02aaa39b223FE8D0A0e5C4F27eAD9083C756Cc2; // WETH
path[1] = token;
uniswapRouter.swapExactETHForTokens{value: msg.value}(
amountOutMin,
path,
msg.sender,
block.timestamp + 300
);
}
}
How This Code is Exploitable
- No slippage protection: If an attacker sees a large swap in the mempool, they can execute a transaction before the user, manipulating the price.
- Publicly visible transactions: Since Ethereum transactions are publicly broadcast before confirmation, bots can detect and front-run them.
Advanced MEV Mitigation Strategies
1. Private Transactions via Flashbots
Flashbots is a research and development organization that provides a private relay network for Ethereum transactions. By sending transactions through Flashbots, they bypass the public mempool, preventing MEV bots from detecting and front-running trades.
How to Use Flashbots
- Developers can send transactions via the Flashbots RPC instead of the public network.
- These transactions remain hidden until mined, eliminating the risk of mempool-based front-running.
2. Implementing MEV-Resistant Order Matching
For DEX developers, designing off-chain order matching or commit-reveal schemes can prevent bots from anticipating trades.
Example of a commit-reveal approach:
contract SecureTrade {
mapping(bytes32 => address) public commitments;
function commitOrder(bytes32 commitment) external {
commitments[commitment] = msg.sender;
}
function revealOrder(uint amount, address token, uint nonce) external {
bytes32 hash = keccak256(abi.encodePacked(amount, token, nonce, msg.sender));
require(commitments[hash] == msg.sender, "Invalid order");
delete commitments[hash];
// Execute trade securely
}
}
3. Using Time-Weighted Average Pricing (TWAP)
Rather than executing a single large trade, TWAP breaks large orders into smaller ones over time, reducing the impact of front-running.
Conclusion: Protect Your Web3 Project from MEV Attacks
MEV is an advanced yet critical problem in Web3 that requires innovative solutions. At Web3Dev, we specialize in securing blockchain applications against MEV threats by implementing:
- Custom transaction relayers to avoid public mempool exposure
- Secure smart contract design with commit-reveal patterns
- Optimization strategies to reduce slippage and prevent front-running
Get Professional Web3 Security Services
Web3 security is complex but essential for any serious blockchain project. Web3Dev provides expert smart contract security audits, MEV-resistant DEX design, and transaction relayer solutions.
🚀 Secure your Web3 project today. Contact us for cutting-edge Web3 security services!
No Comments