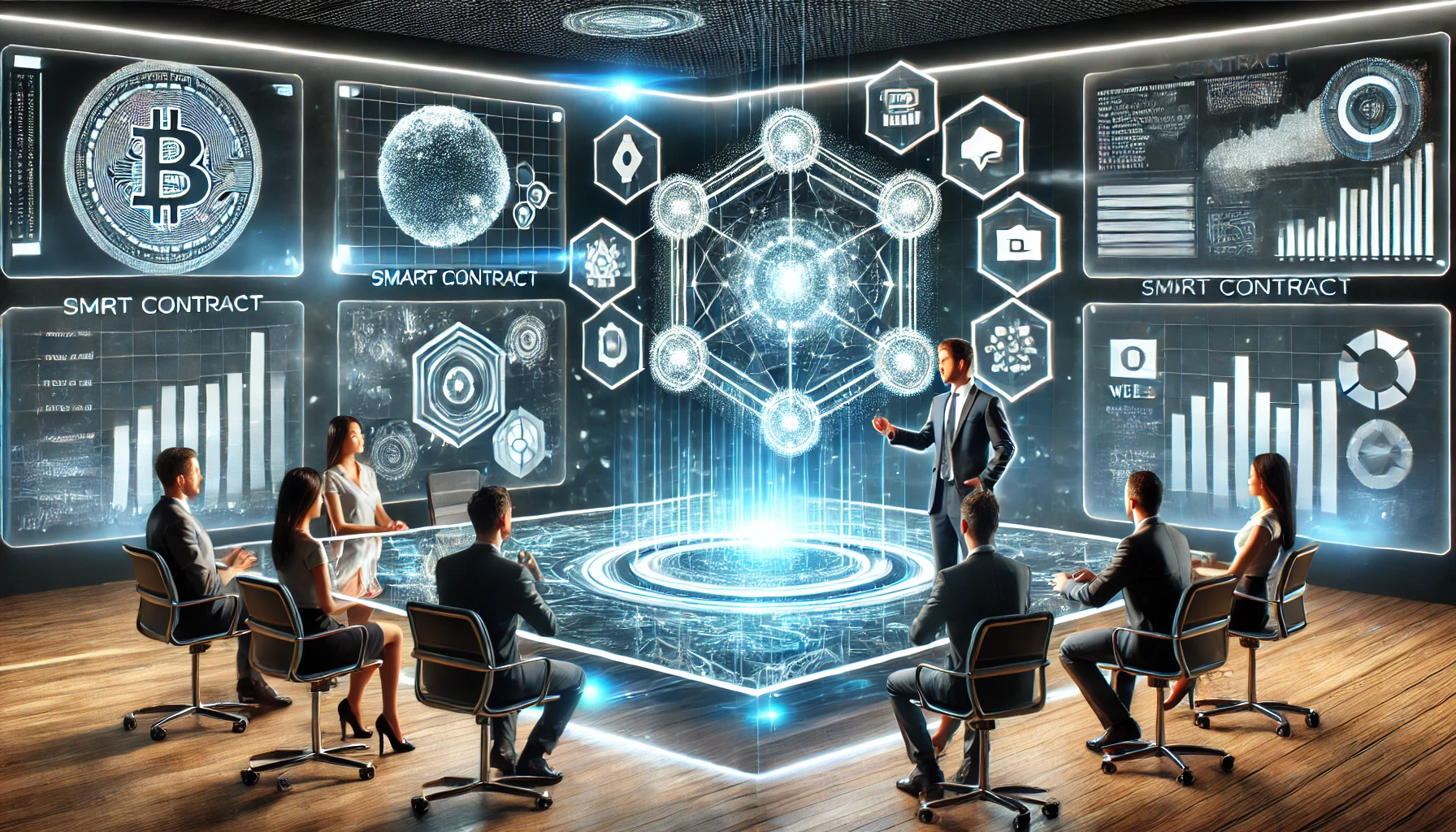
Unrestricted Ether Flow in Smart Contracts: A Common Vulnerability and How to Fix It
Smart contracts are the backbone of decentralized applications (dApps), but they are not immune to vulnerabilities. One often-overlooked issue is unrestricted Ether flow, where a contract allows Ether to be sent or received without proper checks or restrictions. This can lead to unexpected behavior, security risks, and even loss of funds. This article will explain what unrestricted Ether flow is, how it can be exploited, and provide solutions to prevent it. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What is Unrestricted Ether Flow?
Unrestricted Ether flow occurs when a contract allows Ether to be sent or received without proper validation or restrictions. This can happen in several ways, such as:
- Unrestricted
payable
Functions: Functions marked aspayable
can receive Ether, but if they lack proper checks, they may allow unintended transfers. - Unchecked External Calls: Contracts that send Ether to external addresses without validating the recipient or the amount can be exploited.
- Missing Withdrawal Patterns: Contracts that hold Ether but lack proper withdrawal mechanisms can lock funds indefinitely.
How Does Unrestricted Ether Flow Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
function deposit() public payable {
// Accepts Ether without any restrictions
}
function withdraw(uint256 _amount) public {
payable(msg.sender).transfer(_amount); // No access control or checks
}
}
In this contract, the deposit
function accepts Ether without any restrictions, and the withdraw
function allows anyone to withdraw any amount of Ether. This can lead to:
- Unauthorized Withdrawals: An attacker could drain the contract’s balance.
- Locked Funds: If the contract holds Ether but lacks a proper withdrawal mechanism, funds may become inaccessible.
The Impact of Unrestricted Ether Flow
Unrestricted Ether flow can lead to:
- Loss of Funds: Attackers can exploit unrestricted functions to drain the contract’s balance.
- Unexpected Behavior: Ether may be sent to unintended recipients or locked in the contract.
- Reduced Trust: Users may lose confidence in the contract if funds are mishandled.
Solutions to Prevent Unrestricted Ether Flow
1. Implement Access Control
Restrict sensitive functions, such as withdrawals, to authorized users. Use OpenZeppelin’s Ownable
or AccessControl
libraries.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/access/Ownable.sol";
contract Secure is Ownable {
function withdraw(uint256 _amount) public onlyOwner {
payable(msg.sender).transfer(_amount);
}
}
2. Validate Function Parameters
Ensure that function parameters are validated to prevent unauthorized actions. For example, check that the caller is the owner or has the required role.
pragma solidity ^0.8.0;
contract Secure {
address public owner;
constructor() {
owner = msg.sender;
}
function withdraw(uint256 _amount) public {
require(msg.sender == owner, "Unauthorized");
payable(msg.sender).transfer(_amount);
}
}
3. Use Pull Over Push for Withdrawals
Instead of pushing Ether to users, allow them to pull funds themselves. This prevents a single failed transaction from blocking others.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw() public {
uint256 balance = balances[msg.sender];
require(balance > 0, "No balance to withdraw");
balances[msg.sender] = 0;
payable(msg.sender).transfer(balance);
}
}
4. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including unauthorized withdrawals and unexpected Ether transfers. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent vulnerabilities like unrestricted Ether flow.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments