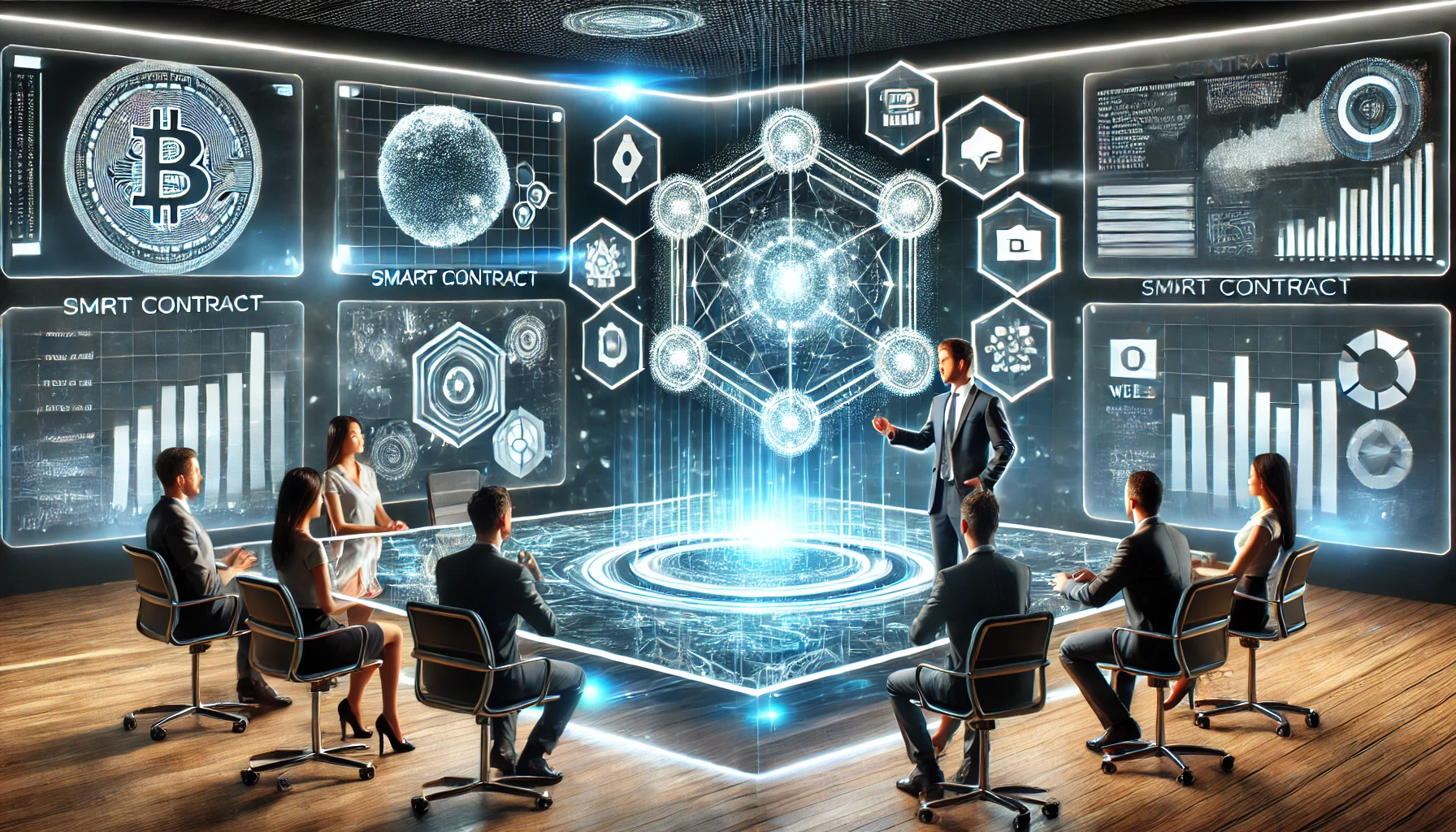
Uninitialized Storage Pointers in Solidity: A Hidden Danger and How to Avoid It
Smart contracts are powerful tools for building decentralized applications (dApps), but they come with their own set of challenges. One often-overlooked issue is the use of uninitialized storage pointers in Solidity. This vulnerability can lead to unexpected behavior, security risks, and even loss of funds. In this article, we’ll explain what uninitialized storage pointers are, how they can be exploited, and provide solutions to avoid them. Additionally, we’ll highlight how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What are Uninitialized Storage Pointers?
In Solidity, storage pointers are references to specific locations in a contract’s storage. If a storage pointer is not explicitly initialized, it may point to an unintended location, leading to unexpected behavior. This issue often arises when developers mistakenly use the storage
keyword instead of memory
for local variables or fail to properly initialize complex data structures.
How Do Uninitialized Storage Pointers Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
struct User {
uint256 balance;
bool isActive;
}
mapping(address => User) public users;
function createUser() public {
User storage newUser; // Uninitialized storage pointer
newUser.balance = 100; // This modifies an unexpected storage slot
newUser.isActive = true;
}
}
In this contract, the newUser
variable is declared as a storage
pointer but is not explicitly initialized. As a result, it points to the first slot in storage (slot 0
), which may contain critical data such as the contract owner or other state variables. Modifying newUser
inadvertently overwrites this data, leading to unintended consequences.
The Impact of Uninitialized Storage Pointers
Uninitialized storage pointers can lead to:
- Data Corruption: Critical state variables may be overwritten, causing the contract to behave unpredictably.
- Security Vulnerabilities: Attackers can exploit this issue to manipulate contract logic or steal funds.
- Loss of Funds: Overwriting storage slots may result in the loss of user balances or other valuable data.
Solutions to Avoid Uninitialized Storage Pointers
1. Always Initialize Storage Pointers
Ensure that storage pointers are explicitly initialized before use. For example:
pragma solidity ^0.8.0;
contract Secure {
struct User {
uint256 balance;
bool isActive;
}
mapping(address => User) public users;
function createUser() public {
User storage newUser = users[msg.sender]; // Properly initialized
newUser.balance = 100;
newUser.isActive = true;
}
}
2. Use memory
for Local Variables
For local variables that do not need to persist in storage, use the memory
keyword instead of storage
. This ensures that the variable is stored temporarily and does not interfere with contract storage.
pragma solidity ^0.8.0;
contract Secure {
struct User {
uint256 balance;
bool isActive;
}
function createUser() public pure returns (User memory) {
User memory newUser; // Stored in memory, not storage
newUser.balance = 100;
newUser.isActive = true;
return newUser;
}
}
3. Be Cautious with Complex Data Structures
When working with arrays, structs, or mappings, ensure that all elements are properly initialized before use. For example:
pragma solidity ^0.8.0;
contract Secure {
struct User {
uint256 balance;
bool isActive;
}
User[] public users;
function addUser() public {
users.push(User({
balance: 100,
isActive: true
}));
}
}
4. Conduct Thorough Testing
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including uninitialized storage pointers.
5. Audit Your Code
Regularly audit your smart contracts using tools like Slither, MythX, or professional auditing services to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We follow best practices and implement robust security measures to protect your dApps from vulnerabilities like uninitialized storage pointers.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential vulnerabilities before deployment.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to ensure your dApps remain secure and up-to-date.
Don’t let vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments