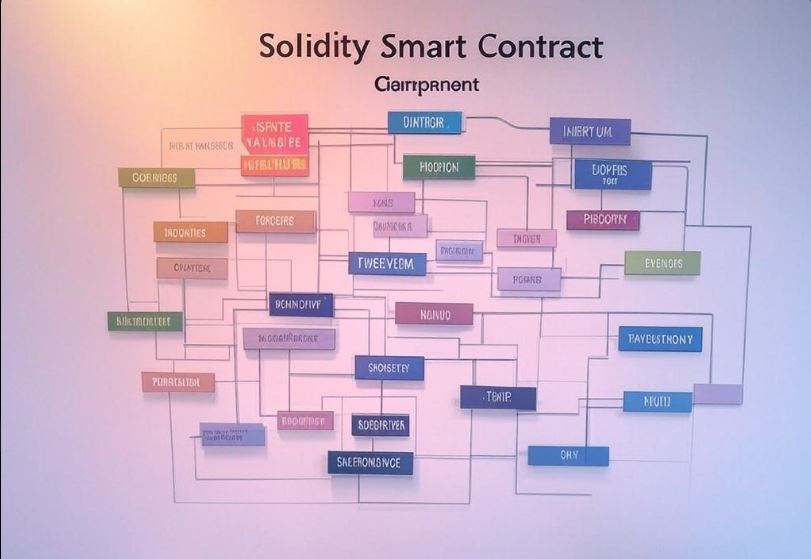
Structure of a Solidity Smart Contract
Solidity is the primary language used for writing smart contracts on Ethereum and other compatible blockchains. A Solidity smart contract is essentially a self-executing piece of code stored within the blockchain that can facilitate, verify, or enforce the negotiation or performance of a contract. Here’s a breakdown of the key components of a Solidity smart contract:
1. Pragma Directive
At the very beginning of every Solidity file, you’ll often see a pragma directive. This specifies the version of the Solidity compiler that your code should use. This is crucial for security and compatibility reasons.
solidity
pragma solidity ^0.8.0;
This directive means the contract should compile with Solidity version 0.8.0 or higher but less than 0.9.0.
2. Contract Declaration
After the pragma, you declare the contract itself using the contract keyword:
solidity
contract MyFirstContract {
// Contract code goes here
}
Everything within these curly braces defines the structure, state, and behavior of the contract.
3. State Variables
State variables are values that persist in the contract’s storage. They’re declared at the top of the contract and can be of various types:
solidity
uint public myUint = 123;
address public owner;
string public myString = "Hello, World!";
- uint for unsigned integers
- address for Ethereum addresses
- string for text data
4. Functions
Functions are where the logic of the contract resides. They can be:
- Public or External: Callable from outside the contract.
- Private or Internal: Only callable from within the contract or derived contracts.
- View or Pure: Functions that don’t modify the state (read-only).
solidity
function setMyUint(uint _myUint) public {
myUint = _myUint;
}
function getMyUint() public view returns (uint) {
return myUint;
}
5. Constructor
The constructor is a special function that gets executed only once when the contract is deployed. It’s used for initializing state variables:
solidity
constructor() {
owner = msg.sender;
}
6. Events
Events allow logging to the blockchain, which can be used by off-chain applications to react to changes in the contract:
solidity
event MyEvent(uint indexed _value, address indexed _from);
function triggerEvent(uint _value) public {
emit MyEvent(_value, msg.sender);
}
7. Modifiers
Modifiers are used to change the behavior of functions in a declarative way:
solidity
modifier onlyOwner() {
require(msg.sender == owner, "Not owner");
_;
}
function changeOwner(address newOwner) public onlyOwner {
owner = newOwner;
}
8. Fallback Function
A fallback function is called when a contract receives Ether without any data (and hence no function specified) or if a function does not exist:
solidity
fallback() external payable {
// Logic for handling Ether received
}
9. Receive Function
Introduced in Solidity 0.6.0, the receive function is used specifically for receiving Ether:
solidity
receive() external payable {
// Logic for handling Ether received
}
Best Practices and Security
- Use visibility specifiers for functions and state variables to control access.
- Implement checks-effects-interactions pattern to prevent reentrancy attacks.
- Use SafeMath or rely on Solidity’s overflow checks in version 0.8.0+.
- Audit your code. Smart contracts are immutable once deployed; thus, extensive testing and auditing are necessary.
Conclusion
A Solidity smart contract’s structure is designed to be both functional and secure, providing a framework where on-chain logic can be executed in a decentralized and trustless manner. Understanding these components is key to writing effective and secure smart contracts. Remember, the simplicity in design can lead to robustness in execution, but always approach with caution due to the immutable nature of blockchain deployments.
No Comments