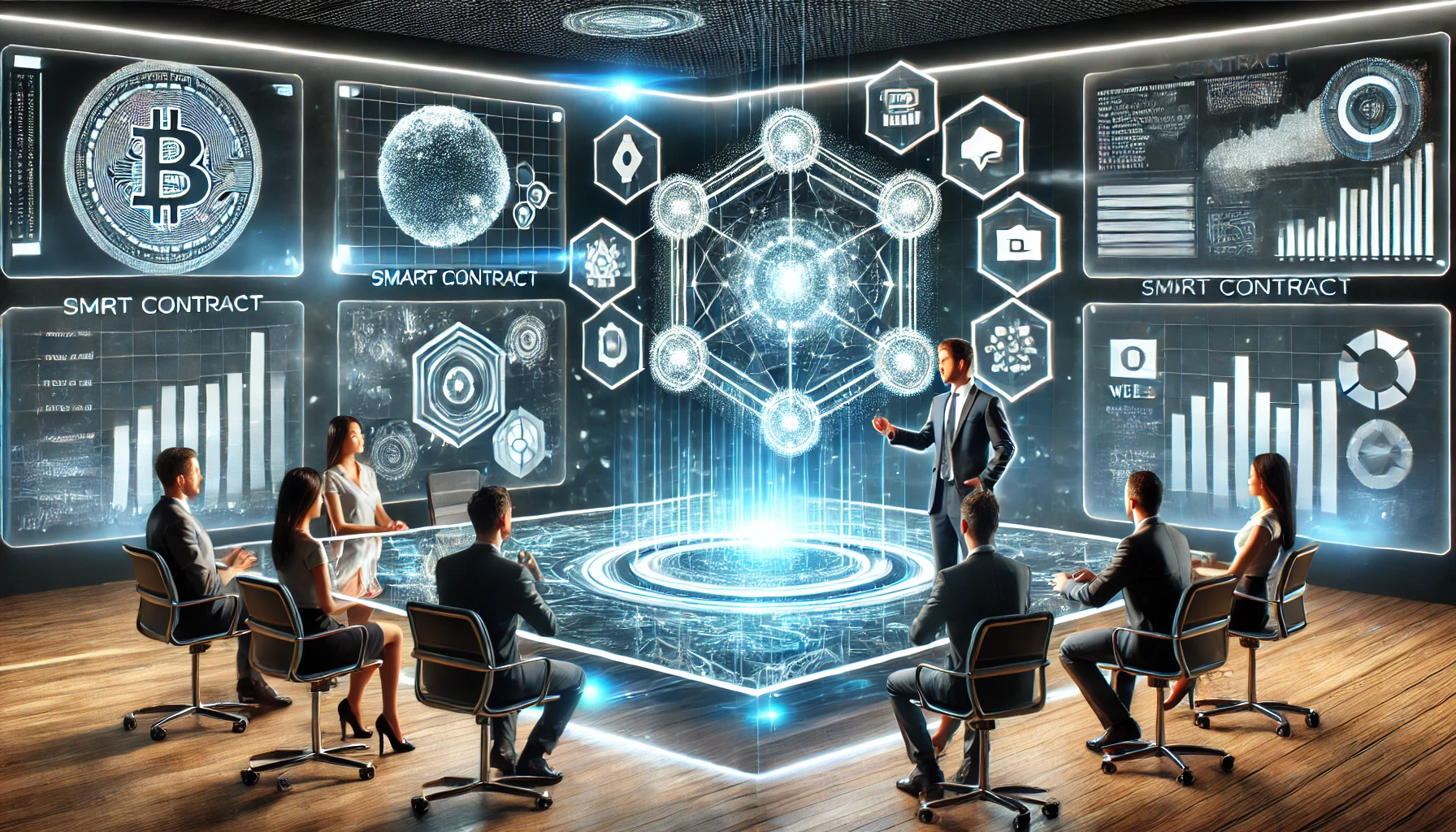
Unchecked External Calls in Smart Contracts: A Common Vulnerability and How to Fix It
Smart contracts are the foundation of decentralized applications (dApps), but they are not immune to vulnerabilities. One of the most common and dangerous issues is unchecked external calls, where a contract interacts with an external address or contract without properly handling potential failures. This article will explain what unchecked external calls are, how they can be exploited, and provide solutions to prevent them. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and reliable decentralized systems.
What are Unchecked External Calls?
In Solidity, external calls to other contracts or addresses (e.g., using call
, send
, or transfer
) can fail for various reasons, such as:
- The target contract reverts the transaction.
- The target address is a contract that runs out of gas.
- The target address is malicious and intentionally causes the call to fail.
If the calling contract does not check the return value of these external calls, it may continue execution under the assumption that the call succeeded, leading to unexpected behavior or vulnerabilities.
How Do Unchecked External Calls Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
mapping(address => uint256) public balances;
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
payable(msg.sender).send(_amount); // Unchecked external call
}
}
In this contract, the withdraw
function uses send
to transfer Ether to the caller. However, send
returns a boolean value indicating whether the transfer succeeded. If the transfer fails (e.g., due to a revert in the recipient’s fallback function), the contract will continue execution without reverting, leaving the caller’s balance reduced but without receiving the funds.
The Impact of Unchecked External Calls
Unchecked external calls can lead to:
- Loss of Funds: Users may lose their balances without receiving the corresponding funds.
- Unexpected Behavior: The contract may continue execution under incorrect assumptions, leading to further vulnerabilities.
- Exploitation by Malicious Contracts: Attackers can exploit unchecked calls to manipulate contract logic or steal funds.
Solutions to Prevent Unchecked External Calls
1. Use transfer
or call
with Proper Checks
The transfer
function automatically reverts on failure, making it safer than send
. However, it is limited to 2300 gas, which may not be sufficient for complex operations. For more flexibility, use call
and check the return value.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
(bool success, ) = payable(msg.sender).call{value: _amount}("");
require(success, "Transfer failed");
}
}
2. Use the Checks-Effects-Interactions Pattern
The Checks-Effects-Interactions (CEI) pattern ensures that state changes are made before interacting with external contracts or addresses. This prevents reentrancy attacks and other vulnerabilities.
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function withdraw(uint256 _amount) public {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount; // Update balance first
(bool success, ) = payable(msg.sender).call{value: _amount}("");
require(success, "Transfer failed");
}
}
3. Use Reentrancy Guards
To further protect against reentrancy attacks (which can be triggered by unchecked external calls), use OpenZeppelin’s ReentrancyGuard
.
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/security/ReentrancyGuard.sol";
contract Secure is ReentrancyGuard {
mapping(address => uint256) public balances;
function withdraw(uint256 _amount) public nonReentrant {
require(balances[msg.sender] >= _amount, "Insufficient balance");
balances[msg.sender] -= _amount;
(bool success, ) = payable(msg.sender).call{value: _amount}("");
require(success, "Transfer failed");
}
}
4. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to write comprehensive unit tests that cover edge cases, including failed external calls. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Secure Smart Contracts: We implement best practices to prevent unchecked external calls and other vulnerabilities.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let unchecked external calls or other vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments