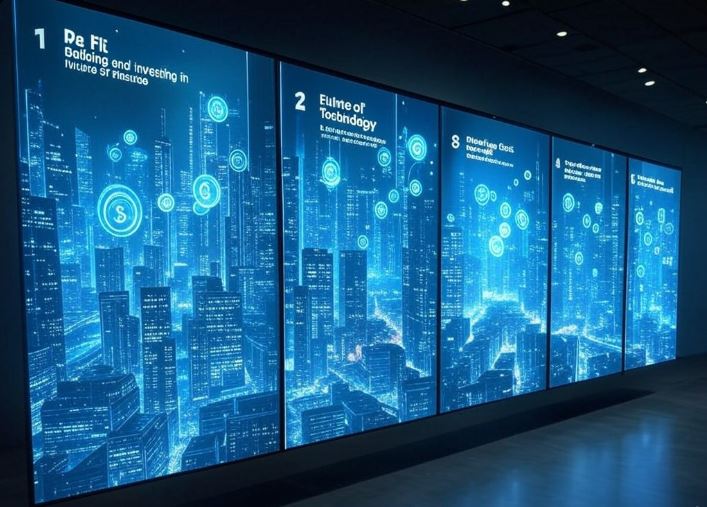
Blockchain Development for Beginners: A Step-by-Step Guide to Building Your First dApp
Introduction
Blockchain technology is the backbone of the Web3 revolution, powering everything from cryptocurrencies to decentralized applications (dApps). If you’re a developer looking to break into this exciting field, you’re in the right place. This guide will walk you through the basics of blockchain development and help you build your first dApp from scratch.
Whether you’re a seasoned programmer or a complete beginner, this step-by-step tutorial will equip you with the knowledge and tools to start creating decentralized solutions. Let’s dive in!
What is Blockchain Development?
Blockchain development involves creating applications and systems that run on a blockchain network. Unlike traditional apps, which rely on centralized servers, dApps operate on a decentralized network of nodes, ensuring transparency, security, and immutability.
Key components of blockchain development include:
- Smart Contracts: Self-executing code that automates transactions and processes.
- Consensus Mechanisms: Protocols like Proof of Work (PoW) or Proof of Stake (PoS) that ensure network agreement.
- Decentralized Storage: Solutions like IPFS for storing data across a distributed network.
Why Learn Blockchain Development?
- High Demand
Blockchain developers are in high demand as companies and startups rush to adopt Web3 technologies. - Innovation
Blockchain opens up new possibilities for creating transparent, secure, and trustless systems. - Financial Opportunities
From building dApps to launching your own token, blockchain development offers numerous ways to monetize your skills. - Future-Proof Career
As Web3 continues to grow, blockchain development will remain a critical skill in the tech industry.
Step 1: Understand the Basics
Before diving into coding, it’s essential to understand the core concepts of blockchain technology:
- Blocks and Chains: Data is stored in blocks, which are linked together in a chronological chain.
- Decentralization: No single entity controls the network; instead, it’s maintained by a distributed network of nodes.
- Immutability: Once data is recorded on the blockchain, it cannot be altered or deleted.
- Cryptography: Ensures the security and integrity of transactions.
Step 2: Choose a Blockchain Platform
There are several blockchain platforms to choose from, each with its own strengths and use cases:
- Ethereum: The most popular platform for dApp development, known for its robust ecosystem and support for smart contracts.
- Binance Smart Chain (BSC): A faster and cheaper alternative to Ethereum, ideal for DeFi projects.
- Polygon (Matic): A Layer 2 scaling solution for Ethereum, offering low fees and high throughput.
- Solana: A high-performance blockchain designed for scalability and speed.
For beginners, Ethereum is a great starting point due to its extensive documentation and developer tools.
Step 3: Set Up Your Development Environment
To start building on Ethereum, you’ll need the following tools:
- Node.js: A JavaScript runtime environment for running your development server.
- Truffle Suite: A development framework for Ethereum that simplifies smart contract development and testing.
- Ganache: A personal blockchain for testing your dApp locally.
- MetaMask: A browser extension wallet for interacting with your dApp.
- Remix IDE: An online tool for writing, testing, and deploying smart contracts.
Step 4: Write Your First Smart Contract
Smart contracts are the building blocks of dApps. Let’s create a simple “Hello World” smart contract using Solidity, Ethereum’s programming language:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
contract HelloWorld {
string public message;
constructor(string memory _message) {
message = _message;
}
function updateMessage(string memory _newMessage) public {
message = _newMessage;
}
}
This contract stores a message and allows users to update it.
Step 5: Deploy and Test Your Smart Contract
- Compile Your Contract: Use Remix IDE or Truffle to compile your Solidity code.
- Deploy to Ganache: Use Ganache to deploy your contract to a local blockchain for testing.
- Interact with Your Contract: Use MetaMask to connect to your local blockchain and interact with your smart contract.
Step 6: Build a Frontend for Your dApp
A dApp isn’t complete without a user interface. Use web development tools like React.js or Vue.js to create a frontend that interacts with your smart contract. Here’s an example using Web3.js, a JavaScript library for Ethereum:
import Web3 from 'web3';
const web3 = new Web3(Web3.givenProvider || "http://localhost:8545");
const contractAddress = "YOUR_CONTRACT_ADDRESS";
const abi = [ /* YOUR_CONTRACT_ABI */ ];
const helloWorldContract = new web3.eth.Contract(abi, contractAddress);
async function updateMessage(newMessage) {
const accounts = await web3.eth.getAccounts();
await helloWorldContract.methods.updateMessage(newMessage).send({ from: accounts[0] });
}
async function getMessage() {
return await helloWorldContract.methods.message().call();
}
Step 7: Deploy Your dApp
Once your dApp is ready, deploy it to a live blockchain network like Ethereum Mainnet or a testnet like Ropsten. Use tools like Infura to connect to the network and deploy your smart contract.
Common Challenges in Blockchain Development
- Gas Fees: High transaction costs can make deploying and using dApps expensive. Optimize your code to reduce gas usage.
- Security Vulnerabilities: Smart contracts are prone to bugs and exploits. Always audit your code and follow best practices.
- Scalability: Blockchain networks can become congested, leading to slow transactions. Explore Layer 2 solutions to improve scalability.
Case Study: Uniswap
Uniswap is a decentralized exchange (DEX) built on Ethereum. Its success lies in its simple yet powerful design:
- Automated Market Making (AMM): Uses smart contracts to provide liquidity and set prices.
- Open Source: Anyone can contribute to or fork the code, fostering innovation and trust.
- Community-Driven: Governed by UNI token holders, ensuring decentralization.
Conclusion
Blockchain development is a rewarding and future-proof skill that opens up endless possibilities in the Web3 ecosystem. By following this guide, you’ve taken the first step toward building your own dApp and contributing to the decentralized future.
At Web3Dev, we’re here to support your journey with tutorials, resources, and a vibrant community of developers. Whether you’re building your first dApp or exploring advanced blockchain concepts, we’ve got you covered.
No Comments