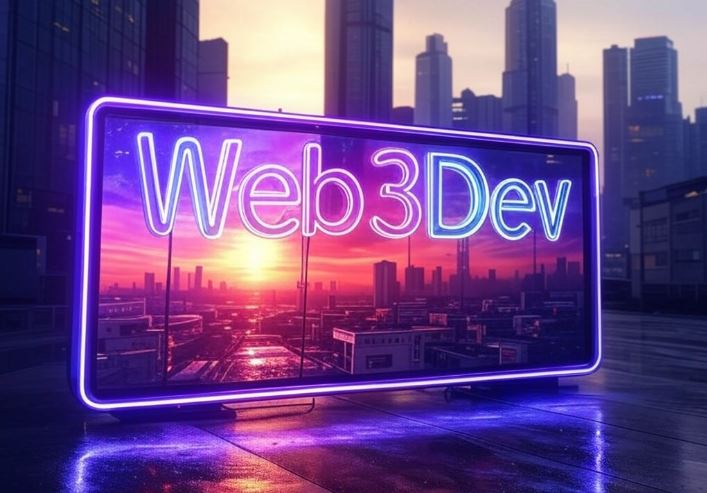
How to Build a Decentralized App (dApp)
Decentralized applications, or dApps, are software applications that run on blockchain networks. Unlike traditional apps, dApps operate in a decentralized manner, meaning they are not controlled by any single entity and leverage the transparency, security, and immutability of blockchain technology. In this guide, we will walk you through the step-by-step process of building your own decentralized app.
Step 1: Understand the Basics of Blockchain and dApps
Before diving into development, it’s crucial to understand what makes dApps different from traditional applications:
- Decentralization: dApps run on a peer-to-peer network rather than centralized servers.
- Smart Contracts: These are self-executing contracts with predefined rules written in code. They automate processes and ensure trust between parties without intermediaries.
- Blockchain: The underlying technology that provides a secure, immutable ledger for storing data and executing smart contracts.
Familiarize yourself with blockchain platforms like Ethereum, Binance Smart Chain, or Polkadot, as these are commonly used to build dApps.
Step 2: Choose the Right Blockchain Platform
Selecting the right blockchain platform is essential for your dApp’s success. Here are some popular options:
- Ethereum: The most widely used platform for dApps, known for its robust smart contract functionality.
- Binance Smart Chain (BSC): Offers lower transaction fees compared to Ethereum, making it a cost-effective alternative.
- Polkadot: Known for its interoperability features, allowing dApps to communicate across multiple blockchains.
- Solana: Known for its high-speed transactions and low fees.
Each platform has its strengths and weaknesses, so choose one based on your project requirements, such as scalability, security, and cost.
Step 3: Set Up Your Development Environment
To start building your dApp, you’ll need to set up your development environment. Here’s how:
- Install Node.js and npm: Most dApp development frameworks require Node.js and npm (Node Package Manager). Download and install them from nodejs.org.
- Install a Code Editor: Use an editor like Visual Studio Code (VS Code), which offers excellent support for blockchain development.
- Install a Blockchain SDK: Depending on the platform you chose, install the appropriate Software Development Kit (SDK). For Ethereum, you can use Truffle or Hardhat.
- Set Up a Wallet: You’ll need a cryptocurrency wallet to interact with the blockchain. MetaMask is a popular choice for Ethereum-based dApps.
- Test Network: Use a test network like Rinkeby (for Ethereum) or Binance Testnet to deploy and test your dApp without spending real cryptocurrency.
Step 4: Write Smart Contracts
Smart contracts are the backbone of any dApp. They define the logic and rules of your application. Here’s how to create them:
- Choose a Programming Language: Solidity is the most common language for writing smart contracts on Ethereum. Other platforms may use different languages (e.g., Rust for Solana).
- Write Your Contract: Start by defining the functions and variables your dApp will need. For example, if you’re building a decentralized voting system, you might have functions like
vote()
,getResults()
, andaddCandidate()
.
// Example: Simple Voting Smart Contract
pragma solidity ^0.8.0;
contract Voting {
mapping(string => uint) public votesReceived;
string[] public candidateList;
constructor(string[] memory candidateNames) {
candidateList = candidateNames;
}
function voteForCandidate(string memory candidate) public {
require(validCandidate(candidate), "Invalid candidate");
votesReceived[candidate] += 1;
}
function totalVotesFor(string memory candidate) public view returns (uint) {
require(validCandidate(candidate), "Invalid candidate");
return votesReceived[candidate];
}
function validCandidate(string memory candidate) private view returns (bool) {
for(uint i = 0; i < candidateList.length; i++) {
if (keccak256(abi.encodePacked(candidateList[i])) == keccak256(abi.encodePacked(candidate))) {
return true;
}
}
return false;
}
}
- Compile and Deploy: Use Truffle or Hardhat to compile and deploy your smart contract to the test network. Ensure that your contract is bug-free and secure before deploying it to the mainnet.
Step 5: Build the Frontend
The frontend of your dApp is what users interact with. It can be built using standard web technologies like HTML, CSS, and JavaScript. Here’s how to connect your frontend to the blockchain:
- Use Web3.js or Ethers.js: These libraries allow your frontend to interact with the blockchain. Install them via npm:
npm install web3
- Connect to MetaMask: Integrate MetaMask into your dApp to allow users to connect their wallets and interact with your smart contracts.
import Web3 from 'web3';
const web3 = new Web3(window.ethereum);
await window.ethereum.enable(); // Request user permission to access their wallet
- Call Smart Contract Functions: Use Web3.js or Ethers.js to call functions in your deployed smart contract. For example, to vote for a candidate:
const contractAddress = "YOUR_CONTRACT_ADDRESS";
const abi = [ /* ABI of your smart contract */ ];
const contract = new web3.eth.Contract(abi, contractAddress);
async function vote(candidate) {
const accounts = await web3.eth.getAccounts();
await contract.methods.voteForCandidate(candidate).send({ from: accounts[0] });
}
- Display Data: Fetch data from the blockchain and display it on your frontend. For example, show the current vote count for each candidate.
Step 6: Test Your dApp
Testing is critical to ensure your dApp works as expected and is free of vulnerabilities. Follow these steps:
- Unit Testing: Write unit tests for your smart contracts using tools like Mocha and Chai (for Truffle) or Waffle (for Hardhat).
- Integration Testing: Test the interaction between your frontend and smart contracts to ensure they work seamlessly.
- Security Audits: Consider hiring a professional auditor to review your smart contracts for vulnerabilities. Tools like MythX and Slither can also help identify potential issues.
Step 7: Deploy Your dApp
Once you’ve thoroughly tested your dApp, it’s time to deploy it to the mainnet:
- Deploy Smart Contracts: Use your deployment script to upload your smart contracts to the mainnet. Be sure to fund your wallet with enough cryptocurrency to cover gas fees.
- Host the Frontend: Host your frontend on a decentralized storage solution like IPFS (InterPlanetary File System) or a traditional web hosting service.
- Publish Your dApp: Share your dApp with the world! Promote it on social media, blockchain forums, and developer communities.
Step 8: Maintain and Update Your dApp
After deployment, your work isn’t over. Regularly monitor your dApp for bugs, performance issues, and security vulnerabilities. If you need to make changes to your smart contracts, consider creating a new version and migrating users to it.
Conclusion
Building a decentralized app (dApp) involves several key steps, from understanding blockchain basics to writing smart contracts, building the frontend, and deploying your application. By following this step-by-step guide, you can create a secure, functional, and user-friendly dApp that leverages the power of blockchain technology.
Remember, the blockchain ecosystem is constantly evolving, so stay updated with the latest trends and best practices to ensure your dApp remains competitive and secure. Happy coding!
Need Help Taking Your Business to the Next Level?
📧 Contact Us | 📅 Book a Meeting
Stay Connected & Get Updates:
🐦 Follow us on X (Twitter)
💬 Join our growing community on Telegram
Let’s build the future together! 🚀
No Comments