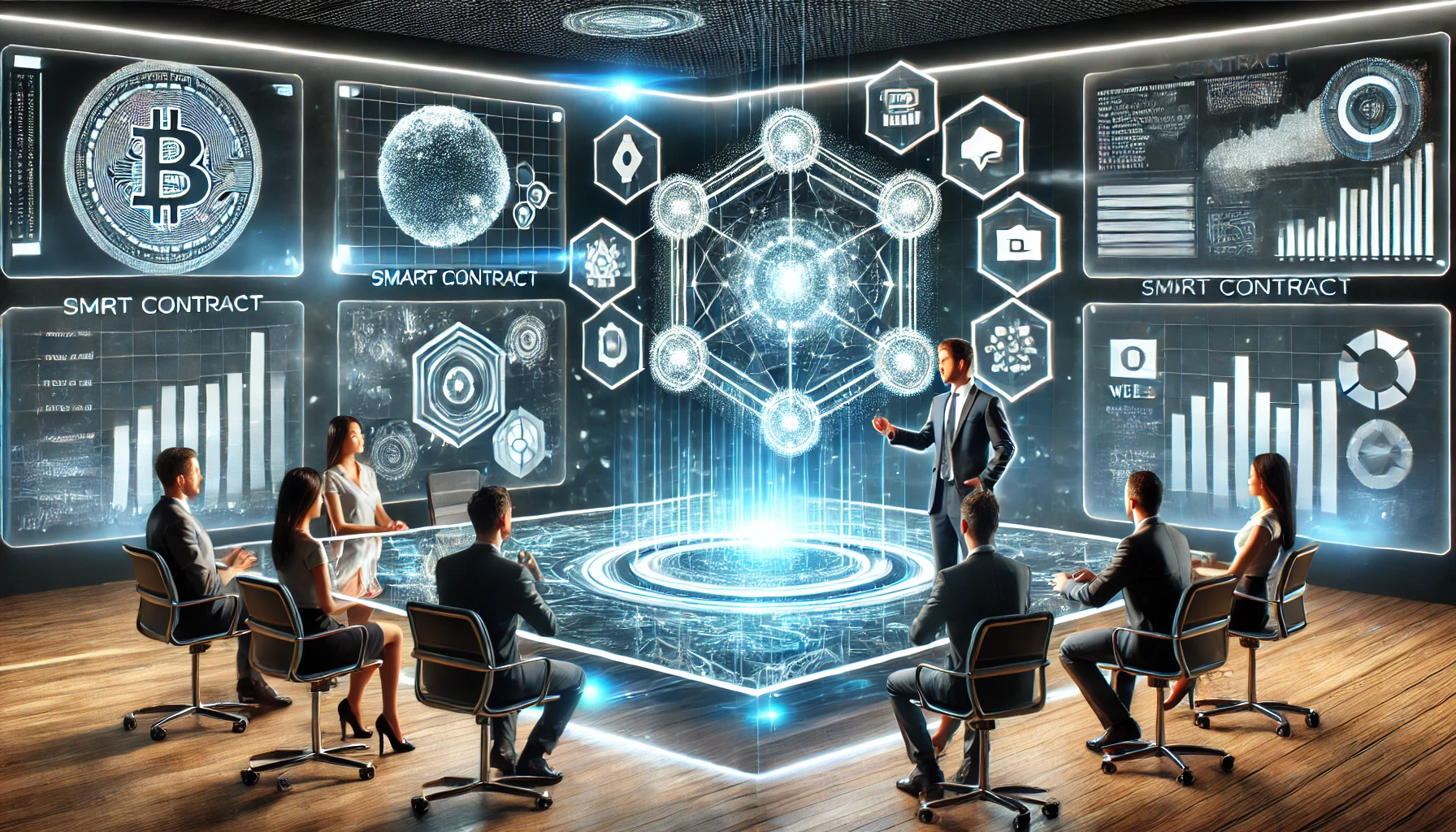
Gas Limit Vulnerabilities in Smart Contracts: A Common Issue and How to Mitigate It
Smart contracts are the backbone of decentralized applications (dApps), but they are not immune to vulnerabilities. One often-overlooked issue is gas limit vulnerabilities, where a contract’s logic can be disrupted or exploited due to the Ethereum network’s gas limit. This article will explain what gas limit vulnerabilities are, how they can be exploited, and provide solutions to mitigate them. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and efficient decentralized systems.
What are Gas Limit Vulnerabilities?
In Ethereum, every transaction requires a certain amount of gas to execute. The network imposes a block gas limit, which restricts the total amount of gas that can be used in a single block. If a transaction exceeds this limit, it will fail. Gas limit vulnerabilities occur when a contract’s logic is designed in a way that can be disrupted or exploited due to these constraints.
Common scenarios include:
- Loops with Unbounded Iterations: Loops that iterate over dynamic arrays or mappings can run out of gas if the data grows too large.
- External Calls: Interactions with external contracts or addresses can consume unpredictable amounts of gas, leading to failures.
- Complex Computations: Expensive operations, such as cryptographic computations or large data manipulations, can exceed gas limits.
How Do Gas Limit Vulnerabilities Work?
Consider the following example of a vulnerable contract:
pragma solidity ^0.8.0;
contract Vulnerable {
address[] public users;
mapping(address => uint256) public balances;
function distributeDividends() public {
for (uint256 i = 0; i < users.length; i++) {
address user = users[i];
uint256 dividend = balances[user] / 10; // Example calculation
(bool success, ) = user.call{value: dividend}("");
require(success, "Transfer failed");
}
}
}
In this contract, the distributeDividends
function iterates over all users and sends them dividends. If the number of users grows too large, the loop may consume more gas than the block limit, causing the transaction to fail. An attacker could exploit this by adding a large number of users to the contract, effectively blocking the distribution of dividends.
The Impact of Gas Limit Vulnerabilities
Gas limit vulnerabilities can lead to:
- Transaction Failures: Critical functions may fail due to exceeding gas limits.
- Denial of Service (DoS): Attackers can exploit gas limits to disrupt contract functionality.
- Loss of Funds: Transactions involving fund transfers may fail, leaving funds stuck in the contract.
Solutions to Mitigate Gas Limit Vulnerabilities
1. Avoid Loops with Unbounded Iterations
Instead of processing all users in a single transaction, use a pull-based mechanism where users claim their funds individually. For example:
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function claimDividend() public {
uint256 dividend = balances[msg.sender] / 10; // Example calculation
require(dividend > 0, "No dividend to claim");
balances[msg.sender] = 0;
payable(msg.sender).transfer(dividend);
}
}
2. Use Pagination for Large Data Sets
If you must process large data sets, break the operation into smaller chunks using pagination. For example:
pragma solidity ^0.8.0;
contract Secure {
address[] public users;
mapping(address => uint256) public balances;
uint256 public currentIndex;
function distributeDividends(uint256 _batchSize) public {
uint256 endIndex = currentIndex + _batchSize;
if (endIndex > users.length) {
endIndex = users.length;
}
for (uint256 i = currentIndex; i < endIndex; i++) {
address user = users[i];
uint256 dividend = balances[user] / 10; // Example calculation
(bool success, ) = user.call{value: dividend}("");
require(success, "Transfer failed");
}
currentIndex = endIndex;
}
}
3. Optimize Gas Usage
Use gas-efficient data structures and algorithms to minimize gas consumption. For example:
- Use
uint256
instead ofuint8
for storage variables (EVM operates on 256-bit words). - Avoid expensive operations like nested loops or complex computations.
4. Test for Gas Limits
Use tools like Truffle, Hardhat, or Foundry to simulate gas usage and test your contract’s behavior under different conditions. For example, test with large data sets to ensure your contract can handle them without exceeding gas limits.
5. Conduct Regular Audits
Regularly audit your smart contracts using tools like Slither, MythX, or professional auditing services to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and innovative Web3 solutions. Our team of blockchain experts can help you:
- Develop Gas-Efficient Contracts: We implement best practices to optimize gas usage and prevent vulnerabilities.
- Conduct Smart Contract Audits: Our thorough auditing process identifies and fixes potential risks in your smart contracts.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let gas limit vulnerabilities or other issues compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments