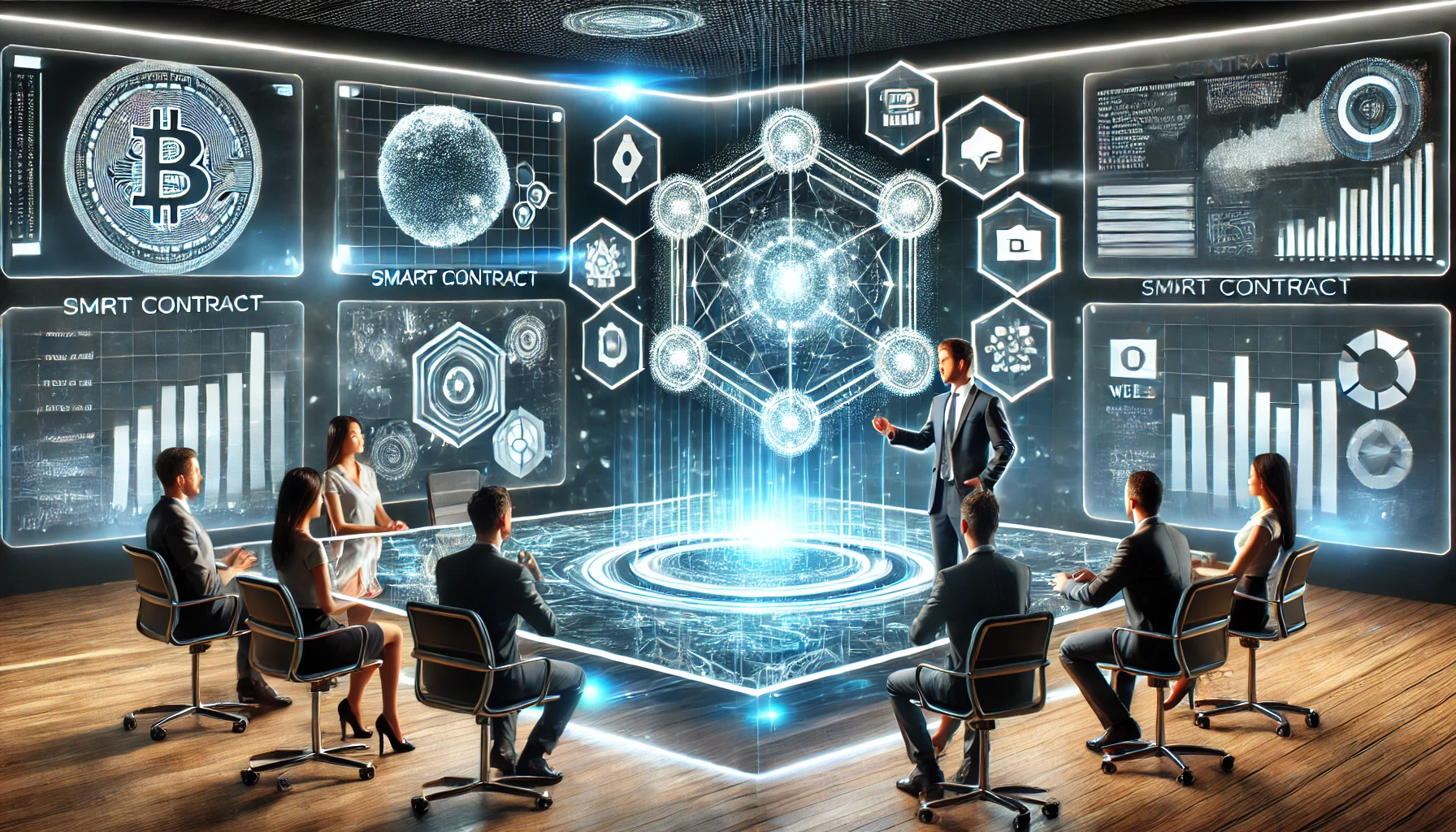
Denial of Service (DoS) Attacks in Smart Contracts: A Common Issue and How to Mitigate It
Smart contracts are a cornerstone of decentralized applications (dApps), but they are not immune to vulnerabilities. One of the most disruptive issues is Denial of Service (DoS) attacks, where an attacker renders a contract unusable or prevents legitimate users from interacting with it. This article will explain how DoS attacks work, their impact, and provide solutions to mitigate them. Additionally, we’ll showcase how Web3Dev, a leading Web3 development agency, can help you build secure and resilient decentralized systems.
What is a Denial of Service (DoS) Attack in Smart Contracts?
A DoS attack in the context of smart contracts occurs when an attacker exploits vulnerabilities to prevent the contract from functioning as intended. This can happen in several ways, such as:
- Blocking Critical Functions: An attacker may exploit logic flaws to block essential functions, making the contract unusable.
- Exhausting Gas Limits: By forcing the contract to perform expensive operations, an attacker can cause transactions to run out of gas and fail.
- Reverting Transactions: An attacker may cause transactions to revert, preventing legitimate users from interacting with the contract.
How Do DoS Attacks Work?
Here are two common scenarios where DoS attacks can occur in smart contracts:
1. Blocking Critical Functions
Consider a contract that allows users to withdraw funds, but processes withdrawals in a loop:
pragma solidity ^0.8.0;
contract Vulnerable {
address[] public users;
mapping(address => uint256) public balances;
function withdraw() public {
for (uint256 i = 0; i < users.length; i++) {
address user = users[i];
uint256 balance = balances[user];
if (balance > 0) {
payable(user).transfer(balance);
balances[user] = 0;
}
}
}
}
In this contract, the withdraw
function iterates through all users and processes their withdrawals. If an attacker adds a large number of users or exploits a vulnerability to prevent the loop from completing, the function may run out of gas or become permanently stuck.
2. Reverting Transactions
Another common issue arises when a contract relies on external calls that can revert. For example:
pragma solidity ^0.8.0;
contract Vulnerable {
mapping(address => uint256) public balances;
function distributeDividends(address[] calldata recipients) public {
for (uint256 i = 0; i < recipients.length; i++) {
address recipient = recipients[i];
uint256 dividend = balances[recipient] / 10; // Example calculation
(bool success, ) = recipient.call{value: dividend}("");
require(success, "Transfer failed");
}
}
}
If one of the recipients is a malicious contract that reverts on receiving Ether, the entire distributeDividends
function will fail, preventing dividends from being distributed to other users.
The Impact of DoS Attacks
DoS attacks can lead to:
- Disruption of Services: Critical functions may become unavailable, rendering the contract unusable.
- Loss of Funds: Users may be unable to withdraw or transfer funds.
- Reputation Damage: Frequent disruptions can erode user trust in your dApp.
Solutions to Mitigate DoS Attacks
1. Avoid Loops with Unbounded Iterations
Instead of processing all users in a single transaction, use a pull-based mechanism where users withdraw their funds individually. For example:
pragma solidity ^0.8.0;
contract Secure {
mapping(address => uint256) public balances;
function withdraw() public {
uint256 balance = balances[msg.sender];
require(balance > 0, "No balance to withdraw");
balances[msg.sender] = 0;
payable(msg.sender).transfer(balance);
}
}
2. Use Pull Over Push for External Calls
Instead of pushing funds to users, allow them to pull funds themselves. This prevents a single failed transaction from blocking others.
3. Implement Circuit Breakers
Add a “circuit breaker” pattern to pause critical functions during an attack or unexpected behavior. For example:
pragma solidity ^0.8.0;
contract Secure {
bool public paused;
mapping(address => uint256) public balances;
modifier whenNotPaused() {
require(!paused, "Contract is paused");
_;
}
function withdraw() public whenNotPaused {
uint256 balance = balances[msg.sender];
require(balance > 0, "No balance to withdraw");
balances[msg.sender] = 0;
payable(msg.sender).transfer(balance);
}
function pause() public {
paused = true;
}
function unpause() public {
paused = false;
}
}
4. Limit Gas Consumption
Avoid operations that consume excessive gas, such as large loops or complex computations. Use gas-efficient data structures and algorithms.
5. Test and Audit Your Code
Use tools like Truffle, Hardhat, or Foundry to simulate DoS scenarios and test your contract’s resilience. Additionally, conduct regular audits to identify and fix vulnerabilities.
How Web3Dev Can Help
At Web3Dev, we specialize in building secure, efficient, and resilient Web3 solutions. Our team of blockchain experts can help you:
- Design Robust Contracts: We implement best practices to prevent DoS attacks and other vulnerabilities.
- Conduct Security Audits: Our thorough auditing process identifies and mitigates potential risks in your smart contracts.
- Optimize Gas Usage: We develop gas-efficient contracts to ensure smooth and cost-effective operations.
- Build Custom dApps: From DeFi platforms to NFT marketplaces, we create tailored solutions to meet your business needs.
- Provide Ongoing Support: We offer maintenance and support services to keep your dApps secure and up-to-date.
Don’t let DoS attacks or other vulnerabilities compromise your Web3 project. Partner with Web3Dev to build with confidence and security.
Contact Web3Dev today to schedule a consultation and take your Web3 project to the next level!
No Comments